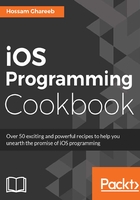
上QQ阅读APP看书,第一时间看更新
How to do it...
- As usual, let's create a new playground named ErrorHandling.
- Let's create now a new error type for a function that will sign up a new user in a system:
enum SignUpUserError: ErrorType{ case InvalidFirstOrLastName case InvalidEmail case WeakPassword case PasswordsDontMatch }
- Now, create the sign up function that throws errors we made in the previous step, if any:
func signUpNewUserWithFirstName(firstName: String, lastName: String, email: String, password: String, confirmPassword: String) throws{ guard firstName.characters.count> 0 &&lastName.characters.count> 0 else{ throw SignUpUserError.InvalidFirstOrLastName } guard isValidEmail(email) else{ throw SignUpUserError.InvalidEmail } guard password.characters.count> 8 else{ throw SignUpUserError.WeakPassword } guard password == confirmPassword else{ throw SignUpUserError.PasswordsDontMatch } // Saving logic goes here print("Successfully signup user") } func isValidEmail(email:String) ->Bool { let emailRegex = "[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}" let predicate = NSPredicate(format:"SELF MATCHES %@", emailRegex) return predicate.evaluateWithObject(email) }
- Now, let's see how to use the function and catch errors:
do{ trysignUpNewUserWithFirstName("John", lastName: "Smith", email: "john@gmail.com", password: "123456789", confirmPassword: "123456789") } catch{ switch error{ case SignUpUserError.InvalidFirstOrLastName: print("Invalid First name or last name") case SignUpUserError.InvalidEmail: print("Email is not correct") case SignUpUserError.WeakPassword: print("Password should be more than 8 characters long") case SignUpUserError.PasswordsDontMatch: print("Passwords don't match") default: print(error) } }