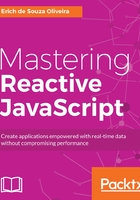
Observables from other sources
The bacon.js has other built-in methods to create observables from common EventStream sources. The objective of this chapter is not to explain all the functions of the bacon.js API. You can check out other possible EventStream sources by referring to bacon.js documentation. The last important method to create an EventStream is the method that lets you create an EventStream from any arbitrary source. This is the fromBinder() method.
The fromBinder() method has the following signature:
Bacon.fromBinder(publisher);
It receives a publisher function as a parameter; this is a function with only one parameter that lets you push events for your EventStream, as illustrated in the following example:
var myCustomEventStream = Bacon.fromBinder(function(push){
push('some value');
push(new Bacon.End());
});
The following code creates an EventStream that emits a string and then finishes the EventStream. As you can see, Bacon.End() is a special object that tells bacon.js when to close the EventStream.
The following example sends more events to the EventStream and prints them to the console:
var myCustomEventStream = Bacon.fromBinder(function(push){
push('some value');
push('other value');
push('Now the stream will finish');
push(new Bacon.End());
});
myCustomEventStream
.onValue((value)=>
console.log(value)
);
If you run this code, you should see the following output:
some value
other value
Now the stream will finish
When we want to propagate an error on our observable, we need to use the Bacon.Error() type to wrap the error. The bacon.js is not capable of automatically encapsulating a thrown exception; you always need to encapsulate it manually. It is also important to notice that when an error occurs, bacon.js doesn't end your observable (but you can configure it). Let's change the previous code to add an error as follows:
var myCustomEventStream = Bacon.fromBinder(function(push){
push('some value');
push('other value');
push(new Bacon.Error('NOW AN ERROR HAPPENED'));
push('Now the stream will finish');
push(new Bacon.End());
});
myCustomEventStream
.onValue((value)=>
console.log(value)
);
It will give you the same output and ignore your error, as you haven't added a handler for errors in this observable (we will see later how to add a handler using the onError() method). But if we want to finish the observable as soon as an error happens, we can call the endOnError() method to our observable, as you can see in this code:
var myCustomEventStream = Bacon.fromBinder(function(push){
push('some value');
push('other value');
push(new Bacon.Error('NOW AN ERROR HAPPENED'));
push('Now the stream will finish');
push(new Bacon.End());
}).endOnError();
myCustomEventStream
.onValue((value)=>
console.log(value)
);
It will give you the following output:
some value
other value