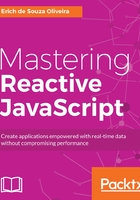
Observables from DOM events (asEventStream)
To create an EventStream from DOM events (a mouse click for instance), we will need an HTML page with jQuery (or Zepto.js); bacon.js adds the asEventStream()method for all jQuery objects. So if we want to create an EventStream from a button click, we can use the following code:
var clickEventStream = $('#myButton').asEventStream('click');
This line creates an EventStream from button clicks on a DOM object with the ID myButton. If we want to execute an action every time this button is clicked, we will need to use the onValue() method from this event stream. The following code shows an alert on the screen every time the user clicks on the button:
clickEventStream.onValue(function(){
alert('Button clicked');
});
This code adds a function to be called every time an event happens in this EventStream object, as it emits an event every time myButton is clicked. The following code will show an alert every time this button is clicked.
As this is your first HTML code, I will paste the full HTML here so you can create a file with it and test it in your own browser:
<html>
<head></head>
<body>
<button id="myButton">CLICK</button>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/bacon.js/0.7.88/Bacon.min.js"></script>
<script>
var clickEventStream = $('#myButton').asEventStream('click');
clickEventStream.onValue(function(){
alert('Button clicked');
});
</script>
</body>
</html>