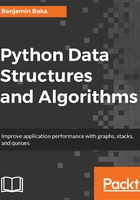
Tuples
Tuples are immutable sequences of arbitrary objects. They are indexed by integers greater than zero. Tuples are hashable, which means we can sort lists of them and they can be used as keys to dictionaries. Syntactically, tuples are just a comma-separated sequence of values; however, it is common practice to enclose them in parentheses:
tpl= ('a', 'b', 'c')
It is important to remember to use a trailing comma when creating a tuple with one element, for example:
t = ('a',)
Without the trailing comma, this would be interpreted as a string.
We can also create a tuple using the built-in function tuple(). With no argument, this creates an empty tuple. If the argument to tuple() is a sequence then this creates a tuple of elements of that sequence, for example:

Most operators, such as those for slicing and indexing, work as they do on lists. However, because tuples are immutable, trying to modify an element of a tuple will give you a TypeError. We can compare tuples in the same way that we compare other sequences, using the ==, > and < operators.
An important use of tuples is to allow us to assign more than one variable at a time by placing a tuple on the left-hand side of an assignment, for example:

We can actually use this multiple assignment to swap values in a tuple, for example:

A ValueError will be thrown if the number of values on each side of the assignment are not the same.