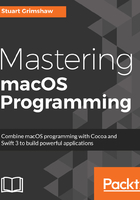
A quick and simple sketch app
Just to give us something to type our experiments into, we will create an extremely simplistic app, one that will present a window into which the user can type a rate of sales tax and a pre-tax price, and will present the resulting price inclusive of sales tax.
It shall be called Sales Tax Calc. I know, it's not a brilliant name.
Create a new Xcode project called SalesTaxCalc, setting it to use Storyboards.
Drag four labels and two standard text fields onto the default View Controller's View object:

The two text fields and the label in the right-hand column will need to be connected to these outlets in the ViewController.swift file:
@IBOutlet weak var rateTextField: NSTextField!
@IBOutlet weak var preTaxTextField: NSTextField!
@IBOutlet weak var resultLabel: NSTextField!
The two text fields need to be connected to these two IBAction methods:
@IBAction func rateDidChange(_ sender: AnyObject){
}
@IBAction func preTaxDidChange(_ sender: AnyObject){
}
The whole code for the ViewController.swift file should look as follows:
import Cocoa
class ViewController: NSViewController
{
@IBOutlet weak var rateTextField: NSTextField!
@IBOutlet weak var preTaxTextField: NSTextField!
@IBOutlet weak var resultLabel: NSTextField!
override func viewDidLoad() {
super.viewDidLoad()
}
override var representedObject: Any? {
didSet {
}
}
@IBAction func rateDidChange(_ sender: AnyObject)
{
calculateFullPrice()
}
@IBAction func preTaxDidChange(_ sender: AnyObject)
{
calculateFullPrice()
}
func calculateFullPrice()
{
let currencyString = "$"
let rate = rateTextField.doubleValue / 100.0 + 1.0
let fullPrice = preTaxTextField.doubleValue * rate
resultLabel.stringValue = currencyString
+ String(format: "%.2f", fullPrice)
}
}
Hit Run to test that the app builds and runs without problems.