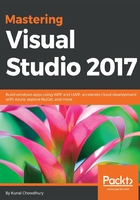
Literal improvements in C# 7.0
There were two types of literals supported in C# prior to C# 7.0. They are decimal literals and hexadecimal literals. For example, 490 is a decimal literal, whereas 0x50A or 0X50A is a hexadecimal literal, equivalent to the decimal value 490. Please note that the prefixes 0x and 0X define the same thing.
Here's an example for you to easily understand how a hexadecimal literal is used in C#:
class Program { static void Main(string[] args) { double height = 490; double width = 1290; double heightInHex = 0x1EA; // equivalent to decimal 490 double widthInHex = 0x50A; // equivalent to decimal 1290 Console.WriteLine("Height: " + heightInHex + " Width: " + widthInHex); } }
Along with C# 7.0, Microsoft added more support to literals, and they have now introduced binary literals in C# to handle the binary value. The binary literals prefixed with 0b or 0B have the same meaning.
In the following example, the decimal value of the binary literal 0b1110110110 is 950:
class Program { static void Main(string[] args) { double height = 950; double width = 5046; double heightInBinary = 0b1110110110; // decimal 950 double widthInBinary = 0b1001110110110; // decimal 5046 Console.WriteLine("Height: " + heightInBinary + " Width: " + widthInBinary); } }
Once you run the preceding code snippet, you will see the following output in console window:
