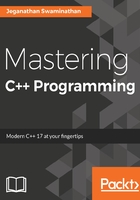
上QQ阅读APP看书,第一时间看更新
Template type auto-deduction for class templates
I'm sure you will love what you are about to see in the sample code. Though templates are quite useful, a lot of people don't like it due to its tough and weird syntax. But you don't have to worry anymore; take a look at the following code snippet:
#include <iostream>
using namespace std;
template <typename T1, typename T2>
class MyClass {
private:
T1 t1;
T2 t2;
public:
MyClass( T1 t1 = T1(), T2 t2 = T2() ) { }
void printSizeOfDataTypes() {
cout << "\nSize of t1 is " << sizeof ( t1 ) << " bytes." << endl;
cout << "\nSize of t2 is " << sizeof ( t2 ) << " bytes." << endl;
}
};
int main ( ) {
//Until C++14
MyClass<int, double> obj1;
obj1.printSizeOfDataTypes( );
//New syntax in C++17
MyClass obj2( 1, 10.56 );
return 0;
}
The preceding code can be compiled and the output can be viewed with the following commands:
g++-7 main.cpp -std=c++17
./a.out
The output of the program is as follows:
Size of t1 is 4 bytes.
Size of t2 is 8 bytes.