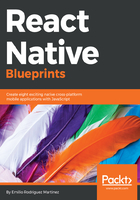
Creating our app's entry point
All React Native apps have one entry file: index.js, we will delegate the root of the component's tree to our src/main.js file:
/*** index.js ***/
import { AppRegistry } from 'react-native';
import App from './src/main';
AppRegistry.registerComponent('rssReader', () => App);
We will also register our app with the operating system.
Now, let's take a look at the src/main.js file to understand how we will set up navigation and start up our component's tree:
/** * src/main.js ***/
import React from 'react';
import { StackNavigator } from 'react-navigation';
import FeedsList from './screens/FeedsList.js';
import FeedDetail from './screens/FeedDetail.js';
import EntryDetail from './screens/EntryDetail.js';
import AddFeed from './screens/AddFeed.js';
import store from './store';
const Navigator = StackNavigator({
FeedsList: { screen: FeedsList },
FeedDetail: { screen: FeedDetail },
EntryDetail: { screen: EntryDetail },
AddFeed: { screen: AddFeed },
});
export default class App extends React.Component {
constructor() {
super();
}
render() {
return <Navigator screenProps={{ store }} />;
}
}
We will use react-navigation as our navigator library and StackNavigator as our navigation pattern. Add each of our screens to the StackNavigator function to generate our <Navigator>. All this is very similar to the navigation pattern we used in Chapter 1, Shopping List, but we incorporated an improvement to it: we are passing store in the screenProps property for our <Navigator>, instead of directly passing the attributes and methods to modify our app's state. This simplifies and cleans up the code base and as we will see in later sections, it will free us from notifying the navigation every time our state changes. All these improvements come for free thanks to MobX.