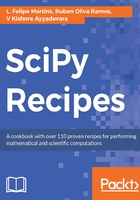
Creating arrays of zeros and ones with a single value
Let's see how to do it all with a single value.
An alternative to empty() is the zeros() function, which creates an array where all elements are initialized to zero, as in the following example:
x = np.zeros((2,3))
The (2,3) tuple argument states that we want a 2 x 3 array of zeros, so we get the following result:
array([[ 0., 0., 0.],
[ 0., 0., 0.]])
Notice that, by default, the data type of the array is float64. If an alternate data type is desired, it must be specified in the call to zeros(), as indicated in the following code:
x = np.zeros((2,3), dtype=np.int64)
This will create a 2 x 3 array of integers.
The ones() function creates an array where all elements are initialized to one, shown as follows:
x = np.ones((4,4))
This command creates a 4 x 4 array of elements of float64 type, all equal to 1. As was the case with the zeros() function, the data type can be specified by setting the dtype argument in the function call.
To create an array where all elements are an arbitrary value, use the full() function, shown as follows:
x = np.full((3,1), -2.5)
This creates a 3 x 1 array with all elements initialized to the value -2.5. The data type of the array will be float64.