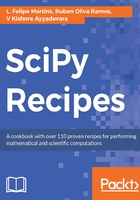
Specifying the data type for elements in an array
To create an array with elements of a given type, specify the dtype option on the array constructor, as follows:
x = np.array([1.2, 3.4, -2.0], dtype=np.float32)
This creates an array of 32-bit floating point values:
array([ 1.20000005, 3.4000001 , -2. ], dtype=float32)
Types of array elements are represented in NumPy by objects from the dtype class, which stands for data type. The following table lists the most commonly used numeric data types available in NumPy:

If a dtype is not explicitly given in the constructor, NumPy will choose the native Python type that can best represent the data. Thus, in a 64-bit architecture, Python floats are converted to float64 and Python integers are usually converted to int64.
Python integers are arbitrary precision integers, that is, they can have an arbitrary number of digits. If a Python integer is too large to be represented as a NumPy integer type, a NumPy object array will be created by default. This is usually not what we want in numerical computation, and in this case it is recommended that you use an explicit type. For example, to create an array with the single 2300 element, represented as a floating point number, use the code:
x = np.array([2**300], dtype=np.float64).