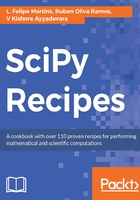
Computing matrix products
When used with NumPy arrays, the * operator represents element by element multiplication, so it cannot be used to compute the matrix product. The matrix product of two arrays is computed with the dot() method of the ndarray object, as demonstrated in the following code:
A = np.array([[2.3, 4.5, -3.1],
[1.2, 3.2, 4.0]])
B = np.array([[ 2.1, -4.6, 0.5, 2.2],
[-1.2, -3.0, 1.7, 3.2],
[ 3.1, 2.6, 1.1, 2.3]])
C = A.dot(B)
In this code segment, we first define two arrays, A and B, with shapes, respectively, of (2,3) and (3,4). Notice that the number of columns of A is equal to the number of rows of B, so that the matrix product AB is well-defined. The matrix product is computed with the statement C = A.dot(B), which outputs the array as follows:
array([[-10.18, -32.14, 5.39, 12.33],
[ 11.08, -4.72, 10.44, 22.08]])
Notice that since A has 2 rows, and B has 4 columns, the product AB is an array of shape (2,4).
Instead of the using the dot() method as suggested previously, we could have used the module-level function np.dot(), writing instead C=np.dot(A,B). The effect is the same, and I tend to prefer using this method because it requires less typing.
The dot() method does the the right thing when computing matrix-vector and vector-matrix products. This is shown in the following examples, where we assume that the array A is the same as in the preceding example:
u = np.array([0.5, 2.3, -4.0])
v = np.array([3.2, -5.4])
w = A.dot(u)
z = v.dot(A)
We first define two one-dimensional arrays, u and v. Then, the w = A.dot(u) statement computes the matrix-vector product Au, and the z = v.dot(A) statement computes the vector-matrix product vA. Notice that, in these computations, u is interpreted as a column vector, and v is interpreted as a row vector. The dot() method in NumPy is smart enough to determine if a one-dimensional should be considered as a row or a column vector. This does not cause any issues, since if the dimensions of the arrays are not compatible, an exception will be raised.
NumPy defines a matrix class, for which the * operator computes matrix products. This class was included for pedagogical reasons only and its use is not recommended in production code. As a matter of fact, I recommend never using the matrix class.