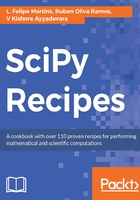
Indexing with Boolean arrays
A Boolean array can be used to select a subset of the items in an array according to a given condition, as illustrated in the following code:
condition = x > 87
y = x[condition]
In this code, we first create the array condition, which is a Boolean array that holds a True value only at the positions that are larger than 87 in the array x. Next, we use the y=x[condition] statement to fetch the elements of x for which the condition is True. The overall effect is to generate an array that contains only the elements in x that are larger than 87, as follows:
array([88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99])
Notice that, when using a Boolean array in this way, the output is always a one-dimensional.
Another common use of a Boolean array index is to select the rows or columns of an array according to a condition. The following example shows you how to select all rows from a two-dimensional array of integers that have an even sum:
x = np.array([[-1, 2, 4], [2, -3, 1], [2, 4, 0],
[-5, 4, 3], [3, 1, 1], [2, 0, -4]])
row_sums = x.sum(axis=1)
y = x[row_sums % 2 == 0, :]
This is how this code works:
- row_sums = x.sum(axis=1) computes the row sums of the array x, storing the results in the row_sums array
- The row_sums % 2 == 0 expression computes a one-dimensional Boolean array with the value True at the indices where row_sums has an even entry, and False otherwise
- Then, x[row_sums % 2 == 0, :] selects the rows of x for which the row sums are even.
After executing the preceding code, the following will be the contents of array y:
array([[ 2, -3, 1],
[ 2, 4, 0],
[-5, 4, 3],
[ 2, 0, -4]])