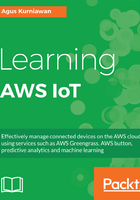
AWS IoT development for Raspberry Pi 3
Raspberry Pi is a famous IoT development. At a low price, you can obtain a minicomputer. Some demo scenarios in this book can be implemented using a desktop computer. Currently, Raspberry Pi 3 is the latest product from Raspberry Pi Foundation. For further information about Raspberry Pi and getting this board, I recommend that you visit the official website at http://raspberrypi.org.
Raspberry Pi 3 can be deployed on various OSes, such as Linux and Windows 10 IoT Core. In this chapter, we will focus on Raspberry Pi 3 with Linux as the OS. We use Raspbian Linux. You can download and install it at https://www.raspberrypi.org/downloads/raspbian/.
Raspbian Linux can work as a console or in desktop mode. You can see Raspbian Linux on Raspberry Pi 3 in the following screenshot:

The corresponding AWS IoT SDK is available at https://aws.amazon.com/iot-platform/sdk/. There are SDKs for Raspberry Pi 3 with the following approaches:
- AWS IoT SDK JavaScript: This is used if you want to develop a program-based JavaScript, such as Node.js
- AWS IoT SDK Python: If you want to develop Python for AWS IoT, you can use this SDK on your Raspberry Pi 3
- AWS IoT SDK Java: For Java developers, this SDK can extend your skill to develop Java applications to connect to AWS IoT
- AWS IoT SDK Embedded C: If you are familiar with C programming, you can choose this SDK to develop an AWS IoT program
Each AWS IoT SDK has a different approach to deploy it on your device. You can follow the guidelines in the documentation for each AWS IoT SDK.
In this section, we use AWS IoT SDK JavaScript on Raspberry Pi 3. The AWS IoT SDK JavaScript installation process is the same as the installation process on a computer. Make sure your Raspberry Pi has Node.js installed. Since Raspbian Linux on Raspberry Pi has Node.js legacy installed, we should uninstall it and then install the latest Node.js. You can uninstall Node.js legacy by typing the following commands:
$ sudo apt-get remove nodered -y
$ sudo apt-get remove nodejs nodejs-legacy -y
$ sudo apt-get remove npm -y
To install the latest Node.js on Raspberry Pi, we can use Node.js from the Nodesource distributor, available at https://github.com/nodesource/distributions. Type the following commands:
$ sudo su -
$ curl -sL https://deb.nodesource.com/setup_8.x | sudo bash -
$ apt-get install nodejs -y
$ node -v
$ npm -v
$ exit
If successful, you should see the latest Node.js and Node Package Manager (NPM), as shown in the following screenshot:

After you have the latest Node.js on Raspberry Pi 3, you can install AWS IoT SDK JavaScript through NPM. This SDK can be found at https://github.com/aws/aws-iot-device-sdk-js. To install this SDK, type the following command on the Terminal of Raspberry Pi 3:
$ npm install aws-iot-device-sdk
For testing, we use the same program from Chapter 1, Getting Started with AWS IoT. You can write this complete program. You should change paths for the certificate, private key, and AWS IoT host. These security certificate files are obtained from AWS IoT. You can read about this in Chapter 1, Getting Started with AWS IoT.
The following is the complete program for our testing:
var awsIot = require('aws-iot-device-sdk');
var device = awsIot.device({
keyPath: 'cert/private.key',
certPath: 'cert/cert.pem',
caPath: 'cert/root-CA.pem',
host: ‘<host-aws-iot-server>',
clientId: 'user-testing',
region: 'ap-southeast-'
});
device
.on('connect', function() {
console.log('connected');
device.subscribe('topic_1');
device.publish('topic_1', JSON.stringify({ test_data: 1}));
});
device
.on('message', function(topic, payload) {
console.log('message', topic, payload.toString());
});
Save this program as the pi-demo.js file.
The screenshot of the preceding code is shown as follows:

Now you can run this program by typing the following command:
$ node pi-demo.js
If we succeed, the program will present a message, as shown in the following screenshot:

You also can verify this by opening the Monitor dashboard from AWS IoT Management Console. You should see a number of connections to your server:
