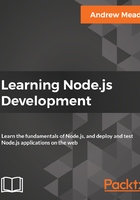
Testing the functionality
The next step in the process will be to test this out by creating a new note. We already have two notes, with a title of secret and a title of secret2 in notes-data.json, let's make a third one using the node app.js command in Terminal. We'll use the add command and pass in a title of to buy and a body of food, as shown here:
node app.js add --title="to buy" --body="food"
This should create a new note, and if I run the command, you can see we don't have any obvious errors:

Inside of our notes-data.json file, if I scroll to the right, we have our brand new note as a title of to buy and a body of food:

So, everything is working as expected even though we've refactored the code. Now, the next thing I want to do inside addNote is take a moment to return the note that's being added, and that will happen right after saveNotes comes back. So we'll return note:
if (duplicateNotes.length === 0) {
notes.push(note);
saveNotes(notes);
return note;
}
This note object will get returned to whoever called the function, and in this case, it will get returned to app.js, where we called it in the if else block of the add command in the app.js file. We can make a variable to store this result and we can call it note:
if (command === 'add')
var note = notes.addNote(argv.title, argv.body);
If note exists, then we know that the note was created. This means that we can go ahead and print a message, like Note created, and we can print the note title and the note body. Now, if note does not exist, if it's undefined, this means that there was a duplicate and that title already exists. If that's the case, I want you to print an error message such as Note title already in use.
Now, inside addNote, if the duplicateNotes if statement never runs, we don't have an explicit call to return. But as you know, in JavaScript, if you don't call return, then undefined automatically is returned. This means that if duplicateNotes.length is not equal to zero, undefined will be returned and we can use that as the condition for our statement.
The first thing I'll do here is to create an if statement, right next to the note variable we defined in app.js:
if (command === 'add') {
var note = notes.addNote(argv.title, argv.body);
if (note) {
}
This will be an object if things went well, and it will be undefined if things went poorly. This code in here is only ever going to run if it's an object. The Undefined result will fail the condition inside of JavaScript.
Now, if the note was created successfully, what we'll do is to print a little message to the screen, using the following console.log statement:
if (note) {
console.log('Note created');
}
If things went poorly, inside the else clause, we can call console.log, and we can print something like Note title taken, as shown here:
if (note) {
console.log('Note created');
} else {
console.log('Note title taken');
}
Now, the other thing that we want to do if things went well is print the notes content. I'll do this by first using console.log to print a couple of hyphens. This will create a little space above my note. Then I can use console.log twice: the first time we'll print the title, I'll add Title: as a string to show you what exactly you're seeing, then I can concatenate the title, which we have access to in note.title, as shown in this code:
if (note) {
console.log('Note created');
console.log('--');
console.log('Title: ' + note.title);
Now, the preceding syntax uses an ES5 syntax; we can swap this out with an ES6 syntax using what we've already talked about: template strings. We'll add Title, a colon, and then we can use our dollar sign with our curly braces to inject the note.title variable, as shown here:
console.log(`Title: ${note.title}`);
Similarly, I'll add note.body after this to print out the body of the note. With this in place, the code should look like:
if (command === 'add') {
var note = note.addNote(argv.title, argv.body);
if (note) {
console.log('Note created');
console.log('--');
console.log(`Title: ${note.title}`);
console.log(`Body: ${note.body}`);
} else {
console.log('Note title taken');
}
Now, we should be able to run our app and see both of the title and body notes printed. In Terminal, I'll rerun the previous command. This will try to create a note with to buy, which already exists, so we should get an error message, and right here you can see Note title taken:

Now, we can rerun the command, changing the title to something else, such as to buy from store. This is a unique note title so the note should get created without any problems:
node app.js add --title="to buy from store" --body="food"

As shown in the preceding output, you can see that we get just that: we have our Note created message, our little spacer, and our title along with the body.
The addNote command is now complete. We have an output when the command actually finishes, and we have all the code that runs behind the scenes to add the note to the data that gets stored in our file.