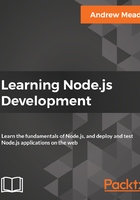
Writing the file in the playground folder
Let's go ahead and comment out all the code we have so far and start with a clean slate. First up, let's go ahead and load in the fs module. The const variable fs will be set equal to require, and we'll pass the fs module that we've used in the past, as shown here:
// var obj = {
// name: 'Andrew'
// };
// var stringObj = JSON.stringify(obj);
// console.log(typeof stringObj);
// console.log(stringObj);
// var personString = '{"name": "Andrew","age": 25}';
// var person = JSON.parse(personString);
// console.log(typeof person);
// console.log(person);
const fs = require('fs');
The next thing we'll do is define the object. This object will be stored inside of our file, and then will be read back and parsed. This object will be a variable called originalNote, and we'll call it originalNote because later on, we'll load it back in and call that variable Note.
Now, originalNote will be a regular JavaScript object with two properties. We'll have the title property, which we'll set equal to Some title, and the body property, which we will set equal to Some body, as shown here:
var originalNote = {
title: 'Some title',
body: 'Some body'
};
The next step that you will need to do is take the original note and create a variable called originalNoteString, and set that variable equal to the JSON value of the object we defined earlier. This means that you'll need to use one of the two JSON methods we used previously in this section.
Now, once you have that originalNoteString variable, we can write a file to the filesystem. I'll write that line for you, fs.writeFileSync. The writeFileSync method, which we used before, takes two arguments. One will be the filename, and since we're using JSON, it's important to use the JSON file extension. I'll call this file notes.json. The other arguments will be text content, originalNoteString, which is not yet defined, as shown in this code block:
// originalNoteString
fs.writeFileSync('notes.json', originalNoteString);
This is the first step to the process; this is how we'll write that file into the playground folder. The next step to the process will be to read out the contents, parse it using the JSON method earlier, and print one of the properties to the screen to make sure that it's an object. In this case, we'll print the title.