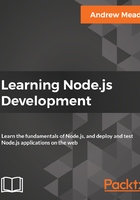
Converting objects into strings
Let's first make a variable called obj, setting it equal to an object. On this object, we'll just define one property, name, and set it equal to your first name; I'll set this one equal to Andrew, as shown here:
var obj = {
name: 'Andrew'
};
Now, let's assume that we want to take this object and work on it. Let's say we want to, for example, send it between servers as a string and save it to a text file. To do this, we'll need to call one JSON method.
Let's take a moment to define a variable to store the result, stringObj, and we'll set it equal to JSON.stringify, as shown here:
var stringObj = JSON.stringify(obj);
The JSON.stringify method takes your object, in this case, the obj variable, and returns the JSON-stringified version. This means that the result stored in stringObj is actually a string. It's no longer an object, and we can take a look at that using console.log. I'll use console.log twice. First up, we'll use the typeof operator to print the type of the string object to make sure that it actually is a string. Since typeof is an operator, it gets typed in lowercase, there is no camel casing. Then, you pass in the variable whose type you want to check. Next up, we can use console.log to print the contents of the string itself, printing out the stringObj variable, as shown here:
console.log(typeof stringObj);
console.log(stringObj);
What we've done here is we've taken an object, converted it into a JSON string, and printed it onto the screen. Over in Terminal, I'll navigate into the playground folder using the following command:
cd playground
We can now use node to run our json.js file. When we run the file, we see two things:

As shown in the preceding code output, first, we will get our type, which is a string, and this is great, because remember, JSON is a string. Next, we will get our object, which looks pretty similar to a JavaScript object, but there are a few differences. These differences are as follows:
- First up, your JSON will have its attribute names automatically wrapped in double quotes. This is a requirement of the JSON syntax.
- Next up, you'll notice your strings are also wrapped in double quotes as opposed to single quotes.
Now, JSON doesn't just support string values, you can use an array, a Boolean, a number, or anything else. All of those types are perfectly valid inside of your JSON. In this case, we have a very simple example where we have a name property and it's set to "Andrew".
This is the process of taking an object and converting it into a string. Next up, we'll define a string and convert that into an object we can actually use in our app.