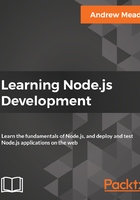
Adding if/else statements
Let's create an if/else block below the console.log('Command: ', command);. We'll add if (command === 'add'), as shown here:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add')
In this case, we'll go through the process of adding a new note. Now, we're not specifying the other arguments here, such as the title or the body (we'll discuss that in later sections). For now, if the command does equal add, we'll use console.log to print Adding new note, as shown in the following code:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
}
And we can do the exact same thing with a command such as list. We'll add else if (command === 'list'), as shown here:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list')
If the command does equal the string list, we'll run the following block of code using console.log to print Listing all notes. We can also add an else clause if there is no command, which is console.log ('Command not recognized'), as shown here:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else {
console.log('Command not recognized');
}
With this in place, we can now rerun our app for a third time, and this time around, you'll see we have the command equal to list, and listing all notes shows up, as shown in the following code:
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else {
console.log('Command not recognized');
}
This means we were able to use our argument to run different code. Notice that we didn't run Adding new note and we didn't run Command not recognized. We could, however, switch the node app.js command from list to add, and in that case, we'll get Adding new note printing, as shown in the following screenshot:

And if we run a command that doesn't exist, for example read, you can see Command not recognized prints as shown in the following screenshot:
