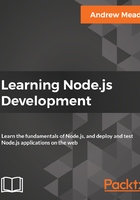
Getting input from the user inside the command line
To start things off, let's run our app from the Terminal. We'll run it pretty similarly to how we ran it in the earlier sections: we'll start with node (I'm not using nodemon since we'll be changing the input), then we'll use app.js, which is the file we want to run, but then we can still type other variables.
If we want to add a note, that might look as a command shown in the following code:
node app.js add
This command will add a note; we can remove a note using the remove command, as shown here:
node app.js remove
And we could list all of our notes using the list command:
node app.js list
Now, when we run this command, the app is still going to work as expected. Just because we passed in a new argument doesn't mean our app is going to crash:

And we actually have access to the list argument already, we're just not using it inside the application.
To access the command-line arguments your app was initialized with, you'll want to use that process object that we explored in the first chapter.
We can log out all of the arguments using console.log to print them to the screen; it's on the process object, and the property we're looking for is argv.
Now save app.js and it'll look like the following:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
console.log(process.argv);
Then we'll rerun this file:

Now, as shown in the preceding command output, we have three items which are as follows:
- The first one points to the executable for Node that was used.
- The second one points to the app file that was started; in this case, it was app.js.
- The third one is where our command-line arguments start to come into play. In it, we have our list showing up as a string.
That means we can access that third item in the array, and that will be the command for our notes application.