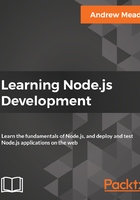
Solution to the exercise
The first step in the process will be to define the new function. In notes.js, I'll set module.exports.add equal to that function, as shown here:
console.log('Starting notes.js');
module.exports.addNote = () => {
console.log('addNote');
return 'New note';
};
module.exports.add =
Let's set it equal to an arrow function. If you used a regular function, that is perfectly fine, I just prefer using the arrow function when I can. Also, inside parentheses, we will be getting two arguments, we'll be getting a and b, as shown here:
console.log('Starting notes.js');
module.exports.addNote = () => {
console.log('addNote');
return 'New note';
};
module.exports.add = (a, b) => {
};
All we need to do is return the result, which is really simple. So we'll enter return a + b:
console.log('Starting notes.js');
module.exports.addNote = () => {
console.log('addNote');
return 'New note';
};
module.exports.add = (a, b) => {
return a + b;
};
Now, this was the first part of your challenge, defining a utility function in notes.js; the second part was to actually use it over in app.js.
In app.js, we can use our function by printing the console.log result with a colon : (this is just for formatting). As the second argument, we'll print the actual results, notes.add. Then, we'll add up two numbers; we'll add 9 and -2, as shown in this code:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const notes = require('./notes.js');
console.log('Result:', notes.add(9, -2));
// var user = os.userInfo();
//
// fs.appendFile('greetings.txt', `Hello ${user.username}! You are ${notes.age}.`);
The result in this case should be 7. If we run the program you can see that we get just that, 7 prints to the screen:

If you were able to get this, congratulations, you successfully completed one of your first challenges. These challenges will be sprinkled throughout the book and they'll get progressively more complex. But don't worry, we'll keep the challenges pretty explicit; I'll tell you exactly what I want and exactly how I want it done. Now, you can play around with different ways to do it, the real goal is to just get you writing code independent of following someone else's lead. That is where the real learning happens.
In the next section, we will explore how to use third-party modules. From there, we'll start building the notes application.