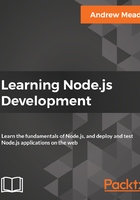
Exporting the functions
Now, obviously, the preceding example is pretty contrived. We'll not be exporting static numbers; the real goal of exports is to be able to export functions that get used inside app.js. Let's take a quick moment to export two functions. In the notes.js file, I'll set module.exports.addnote equal to a function; the function keyword followed by opening and closing parentheses, which is followed by the curly braces:
console.log('Starting notes.js');
module.exports.addNote = function () {
}
Now, throughout the course, I'll be using arrow functions where I can, as shown in the preceding code. To convert a regular ES5 function into an arrow function, all you do is remove the function keyword and replace it with an => sign right between the parentheses and the opening curly braces, as shown here:
console.log('Starting notes.js');
module.exports.addNote = () => {
}
For now though, we'll keep things really simple, using console.log to print addNote. This will let us know that the addNote function was called. We'll return a string, 'New note', as shown here:
console.log('Starting notes.js');
module.exports.addNote = () => {
console.log('addNote');
return 'New note';
};
Now, the addNote function is being defined in notes.js, but we can take advantage of it over in app.js.
Let's take a quick second to comment out both the appendFile and user line in app.js:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const notes = require('./notes.js');
// var user = os.userInfo();
//
// fs.appendFile('greetings.txt', `Hello ${user.username}! You are ${notes.age}.`);
I'll add a variable, call the result, (res for short), and set it equal to the return result from notes.addNote:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const notes = require('./notes.js');
var res = notes.addNote();
// var user = os.userInfo();
//
// fs.appendFile('greetings.txt', `Hello ${user.username}! You are ${notes.age}.`);
Now, the addNote function is a dummy function for the moment. It doesn't take any arguments and it doesn't actually do anything, so we can call it without any arguments.
Then we'll print the result variable, as shown in the following code, and we would expect the result variable to be equal to the New note string:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const notes = require('./notes.js');
var res = notes.addNote();
console.log(res);
// var user = os.userInfo();
//
// fs.appendFile('greetings.txt', `Hello ${user.username}! You are ${notes.age}.`);
If I save both of my files (app.js and notes.js) and rerun things from Terminal, you can see that New note prints to the screen at the very end and just before addNote prints:

This means that we successfully required the notes file we called addNote, and its return result was successfully returned to app.js.
Using this exact pattern, we'll be able to define our functions for adding and removing notes over in our notes.js file, but we'll be able to call them anywhere inside of our app, including in app.js.