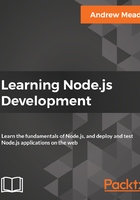
Making a new file to load other files
In the context of our notes app, the new file will store various functions for writing and reading notes. As of now, you don't need to worry about that functionality, as we'll get into the detail later in the section, but we will create the file where it will eventually live. This file will be notes.js, and we'll save it inside the root of our application, right alongside app.js and greetings.txt, as shown here:

For the moment, all we'll do inside notes is to use console.log to print a little log showing the file has been executed using the following code:
console.log('Starting notes.js');
Now, we have console.log on the top of notes and one on the top of app.js. I'll change console.log in the app.js from Starting app. to Starting app.js. With this in place, we can now require the notes file. It doesn't export any functionality, but that's fine.
We'll look at how to export stuff later in the section. For now though, we'll load our module in much the same way we loaded in the built-in Node modules.
Let's make const; I'll call this one notes and set it equal to the return result from require():
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const notes = require('');
var user = os.userInfo();
fs.appendFile('greetings.txt', `Hello ${user.username}!`);
Inside the parentheses, we will pass in one argument that will be a string, but it will be a little different. In the previous section, we typed in the module name, but what we have in this case is not a module, but a file, notes.js. What we need to do is to tell Node where that file lives using a relative path.
Now, relative paths start with ./ (a dot forward slash), which points to the current directory that the file is in. In this case, this points us to the app.js directory, which is the root of our project notes-node. From here, we don't have to go into any other folders to access notes.js, it's in the root of our project, so we can type its name, as shown in the following code:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const notes = require('./notes.js');
var user = os.userInfo();
fs.appendFile('greetings.txt', `Hello ${user.username}!`);
With this in place, we can now save app.js and see what happens when we run our application. I'll run the app using the node app.js command:

As shown in the preceding code output, we get our two logs. First, we get Starting app.js and then we get Starting notes.js. Now, Starting notes.js comes from the note.js file, and it only runs because we required the file inside of app.js.
Comment out this command line from the app.js file, as shown here:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
// const notes = require('./notes.js');
var user = os.userInfo();
fs.appendFile('greetings.txt', `Hello ${user.username}!`);
Save the file, and rerun it from Terminal; you can see the notes.js file never executes because we never explicitly touch it.
We never call it inside Terminal as we do in the preceding example, and we never require.
For now though, we will be requiring it, so I'll uncomment that line.