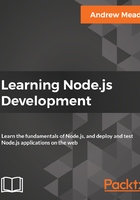
Using template strings
The second way to swap world with user.username in the fs.appendFile is, using an ES6 feature known as template strings. Template strings start and end with the ` (tick) operator, which is available to the left of the 1 key on your keyboard. Then you type things as you normally would.
This means that we'll first type hello, then we'll add a space with the ! (exclamation) mark, and just before !, we will put the name:
console.log('Starting app.');
const fs = require('fs');
const os = require('os');
var user = os.userInfo();
fs.appendFile('greetings.txt', `Hello !`);
To insert a JavaScript variable inside your template string, you use the $ (dollar) sign followed by opening and closing curly braces. Then we will just reference a variable such as user.username:
console.log('Starting app.');
const fs = require('fs');
const os = require('os');
var user = os.userInfo();
fs.appendFile('greetings.txt', `Hello ${user.username}!`);
This is all it takes to use template strings; it's an ES6 feature available because you're using Node v6. This syntax is much easier to understand and update than the string/concatenation version we saw earlier.
If you run the code, it will produce the exact same output. We can run it, view the text file, and this time around, we have Hello Gary! twice, which is what we want here:

With this in place, we are now done with our very basic example and we're ready to start creating our own files for our notes application and requiring them inside app.js in the next section.
First up, you learned that we can use require to load in modules. This lets us take existing functionality written by either the Node developers, a third-party library, or ourselves, and load it into a file so that it can be reusable. Creating reusable code is essential for building large apps. If you have to build everything in an app every time, no one would ever get anything done because they would get stuck at building the basics, things such as HTTP servers and web servers. There are already modules for such stuff, and we'll be taking advantage of the great npm community. In this case, we used two built-in modules, fs and os. We loaded them in using require and we stored the module results inside two variables. These variables store everything available to us from the module; in the case of fs, we use the appendFile method, and in the case of OS, we use the userInfo method. Together, we were able to grab the username and save it into a file, which is fantastic.