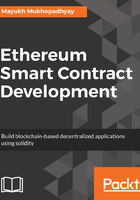
Increment and decrement operations using Solidity
Figure 3.10 shows the code of an increment and decrement operation in Solidity. It also has a function that takes input, and a function, that fetches out this number once the contract gets executed:

Isn't this code a bit more complex than the hello world contract? Let me walk you through this code. The first line starts with pragma. As you have realized already, it just states which version of Solidity we are about to use. In our case, we are using version 0.4.11. As I type, remix IDE supports versions up to 0.4.16.
To understand pragma, let us go back to our brunch buffet analogy from Chapter 2, Grokking Ethereum. If I specify during the buffet reservation that I am a strict vegan, I am giving the buffet organizers some sort of directive, in advance, that I do not eat non-vegan dishes like meat, fish, poultry, or eggs. So, I get introduced to only those counters which serve vegan dishes when the gala brunch begins. Most obviously, I will be annoyed and flag it to the organizers if I am mistakenly served tender lamb kebabs in place of broccoli curry. Then, I would get my plates replaced even before touching or eating the food, no questions asked.
In a similar way, pragma is a language construct that gives some sort of directive to the compiler about how to process its inputs. Generally, a pre-processor handles such language constructs and specifies compiler behavior. In our example, our directive is to make the compiler behave as if it is running a smart contract on Solidity version 0.4.11 or lower.
So, what happens if we do not write this line? Well, nothing bad happens. A warning is thrown, as shown in Figure 3.11, and the code compiles just fine. However, it now uses the default Solidity version, 0.4.16 in our case. By analogy, we can't get angry at the buffet organizers for serving me lamb kebabs if I forget to tell them that I am a vegan. I then somehow manage to eat the food served to me by avoiding the non-vegan portion and save all from further embarrassment.
So, what is the point I am trying to make here? The point I am trying to make is to stick to good programming practice, so that your codes are readable to other programmers who might debug or enhance it in the distant future. As all of the source codes are open source and hosted on GitHub-like platforms, and developers inherently hate to write explicit documentations, it is a recommended professional etiquette to write clean code with logical inline comments:

Let us get back to our code:
- First, we start by defining the contract. We name it ArithValue, as we are going to store and manipulate a value using arithmetic operations. There are no hard-and-fast naming rules here. Try to stick to some logical names.
- Then, we declare an unsigned integer value that will store our default number, which must be a positive integer.
- Now we need to give our contract a constructor function. So, what is a constructor function? Basically, this is a function that will be called whenever our contract is deployed and initiated for the first time. What's special about this constructor function is that it has the same name as that of the contract, that is, ArithValue. Inside the constructor function, we assign 100 to the number variable. So, now, whenever the contract gets initiated for the first time, it will set a default value of 100 to our unsigned integer variable number.
- Now we create four other functions inside the contract that basically set, fetch, increment, and decrement the value we assigned to the variable number. These steps will be shown in Figure 3.12, Figure 3.13, Figure 3.14, and Figure 3.15, after we create the contract. Ideally, we must first publish the contract and then create it to instantiate, which will cost us real ethers on a blockchain, but at this point the publishing is of little sense, as we are working on a simulated blockchain environment and will not publish anything on a permanent basis.
- As we can see in the Figure 3.12, once the contract is deployed and instantiated, four functions, namely fetchNumber, decrementNumber, incrementNumber, and setNumber, get created. Due to the constructor function, our default value is set to 100 in the contract.
- As depicted in Figure 3.13, if we press the incrementNumber button and press the fetchNumber button, the value gets incremented to 101.
- In Figure 3.14, we type in a random positive integer 143 and press setNumber.
- We then press fetchNumber and our value has changed to 143.
- Then, as shown in Figure 3.15, we press the decrementNumber button, followed by fetchNumber; the value 143 is decremented to 142.
As you try this example on your laptop, keep a watch on the Account drop-down tab, which is just below the Environment drop-down tab. Did you notice the decreasing value of the ether? Do check it:



