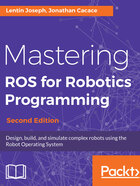
Adding custom msg and srv files
In this section, we will look at how to create custom messages and services definitions in the current package. The message definitions are stored in a .msg file and the service definitions are stored in a .srv file. These definitions inform ROS about the type of data and name of data to be transmitted from a ROS node. When a custom message is added, ROS will convert the definitions into equivalent C++ codes, which we can include in our nodes.
We can start with message definitions. Message definitions have to be written in the .msg file and have to be kept in the msg folder, which is inside the package. We are going to create a message file called demo_msg.msg with the following definition:
string greeting int32 number
Until now, we have worked only with standard message definitions. Now, we have created our own definitions and can see how to use them in our code.
The first step is to edit the package.xml file of the current package and uncomment the lines <build_depend>message_generation</build_depend> and <exec_depend>message_runtime</exec_depend>.
Edit the current CMakeLists.txt and add the message_generation line, as follows:
find_package(catkin REQUIRED COMPONENTS roscpp rospy std_msgs actionlib actionlib_msgs message_generation )
Uncomment the following line and add the custom message file:
add_message_files( FILES demo_msg.msg ) ## Generate added messages and services with any dependencies listed here generate_messages( DEPENDENCIES std_msgs actionlib_msgs )
After these steps, we can compile and build the package:
$ cd ~/catkin_ws/ $ catkin_make
To check whether the message is built properly, we can use the rosmsg command:
$ rosmsg show mastering_ros_demo_pkg/demo_msg
If the content shown by the command and the definition are the same, the procedure is correct.
If we want to test the custom message, we can build a publisher and subscriber using the custom message type named demo_msg_publisher.cpp and demo_msg_subscriber.cpp. Navigate to the mastering_ros_demo_pkg/1 folder for these code.
We can test the message by adding the following lines of code in CMakeLists.txt:
add_executable(demo_msg_publisher src/demo_msg_publisher.cpp) add_executable(demo_msg_subscriber src/demo_msg_subscriber.cpp) add_dependencies(demo_msg_publisher mastering_ros_demo_pkg_generate_messages_cpp) add_dependencies(demo_msg_subscriber mastering_ros_demo_pkg_generate_messages_cpp) target_link_libraries(demo_msg_publisher ${catkin_LIBRARIES}) target_link_libraries(demo_msg_subscriber ${catkin_LIBRARIES})
Build the package using catkin_make and test the node using the following commands.
- Run roscore:
$ roscore
- Start the custom message publisher node:
$ rosrun mastering_ros_demo_pkg demo_msg_publisher
- Start the custom message subscriber node:
$ rosrun mastering_ros_demo_pkg demo_msg_subscriber
The publisher node publishes a string along with an integer, and the subscriber node subscribes the topic and prints the values. The output and graph are shown as follows:

The topic in which the nodes are communicating is called /demo_msg_topic. Here is the graph view of the two nodes:

Next, we can add srv files to the package. Create a new folder called srv in the current package folder and add a srv file called demo_srv.srv. The definition of this file is as follows:
string in --- string out
Here, both the Request and Response are strings.
In the next step, we need to uncomment the following lines in package.xml as we did for the ROS messages:
<build_depend>message_generation</build_depend> <exec_depend>message_runtime</exec_depend>
Take CMakeLists.txt and add message_runtime in catkin_package():
catkin_package( CATKIN_DEPENDS roscpp rospy std_msgs actionlib actionlib_msgs message_runtime )
We need to follow the same procedure in generating services as we did for the ROS message. Apart from that, we need additional sections to be uncommented, as shown here:
## Generate services in the 'srv' folder add_service_files( FILES demo_srv.srv )
After making these changes, we can build the package using catkin_make and using the following command, we can verify the procedure:
$ rossrv show mastering_ros_demo_pkg/demo_srv
If we see the same content as we defined in the file, we can confirm it's working.