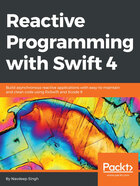
JSON encoding and decoding
Everyone's favorite change to Swift 4 is of course the latest way to parse JSON. If you have been part of the Swift community for a while now, you'll know that every month we have a new hot way to parse JSON or so it feels, and now finally, we have an official way, so let's take a look. Let's talk about the Why first; Swift didn't ship with any native archival or serialization APIs and instead benefited from the Objective-C implementations available to it. While this allowed us to achieve certain end goals, it was both restrictive in that only NSObject subclasses can benefit, and we had to implement solutions for value types and as always, the existing solutions, NSJSONSerialization and so on, are far from Swift like.
The goal, therefore, for this proposal was to allow native archival and serialization of Swift Enums and Structs and to do that in a type safe way. Let's introduce some sample JSON to our playground to see how it works in practice and as an added benefit, we get to work with Swift 4's multiline String literal syntax:
import Foundation
let exampleJson = """
{
"name": "Photography as a Science",
"release_date": "2017-11-12T14:00:00Z",
"authors": [
{
"name": "Noor Jones"
},
{
"name": "Larry Page"
}
]
}
"""
We cannot convert this raw JSON in a datatype, so let's say as follows:
let json = exampleJson.data(using: .utf8)!
Now, let's define a type, and we will keep it simple so that we can see how the new API works:
struct Book {
let name: String
}
We want to decode the JSON into an instance of this struct Book and doing that in Swift 4 is super easy. First, we will make our model conform to our new Codable protocol, after which all we need to do is the following:
struct Book: Codable{
let name: String
}
let photographyBook = try! JSONDecoder().decode(Book.self, from: json)
If we match the values on the instance, we can see that they match the value in the JSON data and that's it, easy isn't it?