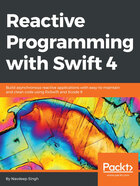
Changes/improvements in Dictionary
Many proposals were made to enhance the Dictionaries and make them more powerful. In certain scenarios, Dictionaries might behave in an unexpected manner, and for this reason, many suggestions were made to change the way Dictionaries currently work in certain situations.
Let’s take a look at an example. Filtering returns the same data type; in Swift 3, if you used a filter operation on a Dictionary, the return type of the result would be a tuple (with key/value labels) and not a Dictionary. Consider this example:
let people = ["Tom": 24, "Alex": 23, "Rex": 21, "Ravi": 43]
let middleAgePeople = people.filter { $0.value > 40 }
After the execution, you cannot use middleAgePeople["Ravi"] since the returned result is not a Dictionary. Instead, you have to follow the tuple syntax to access the desired value because the return type is tuple, middleAgePeople[0].value, which is not implicitly expected.
Thanks to the new release, the current scenario has now changed as the new return type is a Dictionary. This will break any existing implementation in which you had written your code based on the return type, expecting it to be a tuple.
Similarly, while working with Dictionaries, the map() operation never worked the way most developers expected, since the return type could be a single value while you passed in a key-value tuple. Let's look at the following example:
let ages = people.map { $0.value * 2 }
This remains the same in Swift 4, but there is the addition of a new method mapValues(), which will prove to be of more use as it allows values passed to the method to be transformed and spit out as a Dictionary with original keys.
For example, the following code will round off and convert all the given ages to Strings, place them into a new Dictionary with the exact same keys, that is, Tom, Alex, Rex, and Ravi:
let ageBrackets = people.mapValues { "\($0 / 10) 's age group" }
Mapping Dictionary keys is not safe as we might end up creating duplicates.