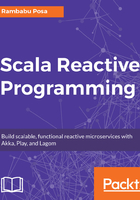
上QQ阅读APP看书,第一时间看更新
For-comprehensions
Scala supports a very rich For loop construct known as For-comprehension. We can use it like Java's extended For loop, as shown here:
scala> val numList = List(1,2,3,4,5) numList: List[Int] = List(1, 2, 3, 4, 5) scala> for(num <- numList) yield num+1 res7: List[Int] = List(2, 3, 4, 5, 6)
When we observe the preceding example, we realize that For-comprehension results in the same container or object with a new set of elements.
For-comprehension = filter + map
We can use For-comprehension to replace the operation that uses both filter and map, as shown here:
scala> val numList = List(1,2,3,4,5) numList: List[Int] = List(1, 2, 3, 4, 5) scala> numList.filter(a => a %2 == 0).map(b => b*b) res8: List[Int] = List(4, 16) scala> for(num <- numList if num % 2 == 0) yield num*num res10: List[Int] = List(4, 16)
Here, For-comprehension is picking one element at a time from numList, checking the condition num % 2 == 0 (even number) and performing the square root operation, that is, num*num.
A combination of these two Higher-Order Functions, that is, filter and map, also does the same.