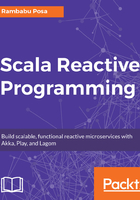
Pattern matching
In Scala, pattern matching is one of the powerful FP constructs, which is not available in many other languages, such as Java. It is used to match an expression with a sequence of alternatives and get the required results.
It is something similar to Java's Switch statement, but it does more. One of the greatest benefits of this FP construct is that we can do matching on almost everything, such as values, objects, Case classes, user-defined classes, and more.
The following syntax defines how to use Scala's match statement to do pattern matching:
[Expression] match {
case [Pattern1] => [A block of code-1] or [Result-1]
case [Pattern2] => [A block of code-1] or [Results2]
...
case [Pattern-n] => [A block of code-1] or [Results-n]
}
As shown in the preceding code snippet, a pattern matching construct starts with an expression E, followed by the match keyword and a set of case blocks. A case consists of the case keyword followed by a pattern, then followed by the => symbol, and finally expression(s) (or a block of code) to be executed, if this pattern matches.
Here, the => symbol separates the pattern from the result, as shown in this example:
scala> val num:Int = 10 num: Int = 10 scala> num match { | case 1 => println("one") | case 10 => println("ten") | } ten
Here, num is an expression and has two case blocks. Each case is printing a value, but not returning anything.
Pattern matching can optionally have a guard clause on the left-hand side of a case, as shown here:
scala> num match { | case x if x % 2 == 0 => println("Even number.") | case _ => println("Odd number.") | }
In the preceding code example, we are using the _ placeholder to match everything and avoid MatchError:
scala> num match { | case x if x % 2 != 0 => println("Odd number.") | } scala.MatchError: 10 (of class java.lang.Integer)
As this match expression is missing the Checking of Even numbers or Default clause, it throws a MatchError. In real-time projects, it is always recommended you use one of the default cases to avoid this kind of error.
It is always recommended you use pattern matching expressions, instead of isInstanceOf/asInstanceOf methods. We will discuss how to pattern match on case classes in the coming sections.
Scala's pattern matching follows the Visitor Design pattern.