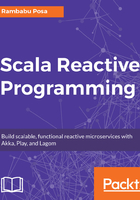
Scala functions
In general, a function is an operation that takes some inputs, processes them, and returns results. It is the same for the Scala language too. Take a look at the following diagram:

Sometimes, a function is also known as an operation, routine, procedure, or method, depending on the context in which we use it.
In Scala, we can define a function using def, as shown here:
def add (a: Int, b: Int) : Int = a + b
Here, the add function has two inputs, a and b, of the same type, Int, and returns Int as a result.
Scala supports some syntactic sugar to write the same function in different ways:
def add (a: Int, b: Int) : Int = { a + b }
If our function has one and only one expression, then we can omit the curly braces; they are optional:
def add (a: Int, b: Int) = a + b
We can omit the result type of Int here. The Scala compiler will guess or infer the return type as follows:
scala> def add (a: Int, b: Int) = a + b add: (a: Int, b: Int)Int
This is known as type inference. This is the beauty of the Scala language feature.
Functions always return only one value.
In Scala, avoid using return to return a function result, as shown here:
scala> def add (a: Int, b: Int) = return a + b <console>:10: error: method add has return statement; needs result type def add (a: Int, b: Int) = return a + b ^
If we use the preceding result type, then the Scala compiler cannot infer its return type. We should manually specify its return type, as shown here:
scala> def add (a: Int, b: Int):Int = return a + b add: (a: Int, b: Int)Int
In Scala, if a function has more than one expression in its body, then we should use curly braces. In this case, curly braces are mandatory:
scala> def add (a: Int, b: Int) = { | val result = a + b | result | } add: (a: Int, b: Int)Int
Here, the add() function is using curly braces because its body has more than one expression. When we don't use return in a function body, the Scala compiler will take the last expression from a function body, evaluate it, and infer that function result type, based on that last expression value.
In the preceding add() function, the Scala compiler evaluates result (the last expression from the function body) and infers its result type as Int.