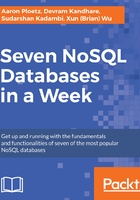
Applying conditional and logical operators on the filter parameter
We can apply conditional parameters while retrieving data from collections, such as IN, AND, and OR with less than and greater than conditions:
- Apply the IN condition: We can set filter parameters so the query can match values from a given set and retrieve values from collections where documents match values from a given set. The syntax for the in parameter is the following:
{<field 1> : {<operator 1> : <value 1>},....}
The following query will return all the documents from user_profiles where the first name is John or Kedar:

Here, we get all the documents with a firstName of either John or Kedar:

The preceding operation corresponds to the following SQL operation query:
SELECT * FROM user_profiles WHERE firstName in('John', 'Kedar');
- Apply the AND condition: If the implicit query applies to more than two fields, it covers the AND condition and does not need to apply it separately. This compound query matches all the conditions specified and returns documents where all these conditions are met.
For example, the following query returns the result where the firstname matches John and the age of the user is less than 29:

This corresponds to the following SQL query:
SELECT * FROM user_profiles WHERE firstName='John' AND age<30;
- Apply the OR condition: Using the $or operator, we can specify the compound query that can apply to two or more fields. The following is the query example where we retrieve any user's profile that has either the first name of John or an age value of less than 30:

This corresponds to the following SQL query:
SELECT * FROM user_profiles WHERE firstName='John' or age<30;
- Apply the AND/OR condition in the combine. The following is a query example where we apply both the AND and OR operators in the combine to retrieve documents. This query returns user's profiles where the firstName is John and the age is less than 30 or the lastName starts with s:

This operation corresponds to the following SQL operation:
SELECT * FROM user_profiles WHERE firstName='John'
AND age<30 OR lastName like 's%';
MongoDB also supports $regex to perform string-pattern matching. Here is a list of the comparison query operators:
Operator |
Description |
$eq |
Matches values that equals a specified value |
$gt |
Matches values that are greater than a specified value |
$gte |
Matches values that are greater than or equal to a specified value |
$lt |
Matches values that are less than a specified value |
$lte |
Matches values that are less than or equal to a specified value |
$ne |
Matches values that are not equal to a specified value |
$in |
Matches values that are specified in a set of values |
$nin |
Matches values that are not specified in a set of values |
Here is a list of logical operators:
Operator |
Description |
$or |
Joins query clauses with the OR operator and returns all the documents that match any condition of the clause. |
$and |
Joins query clauses with the AND operator and returns documents that match all the conditions of all the clauses. |
$not |
Inverts the effects of the query expression and returns all the documents that do not match the given criteria. |
$nor |
Joins query clauses with the logical NOR operator and returns all the documents that match the criteria specified in the clauses. |
MongoDB also uses the findOne method to retrieve documents from the mongo collection. It internally calls the find method with a limit of 1. findOne matches all the documents with the filter criteria and returns the first document from the result set.