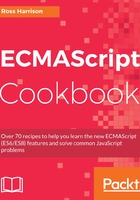
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open your command line application and navigate to the directory containing the 02-creating-client-bundles package.
- Start the Python HTTP server.
- Update the main.js file to use the Array.prototype.values method, and use for..of to loop over the resulting iterator:
import { atlas, saturnV } from './rockets/index.js' export function main () { const rockets = [saturnV, atlas];
for (const rocket of rockets.values()) {
rocket.launch();
} }
- Install the Babel Polyfill package:
npm install --save babel-polyfill
- To shim the bundle, update the webpack.config.js file to add Babel Polyfill to the entry point:
const path = require('path'); module.exports = { entry: ['babel-polyfill', './index.js'], output: { filename: 'bundle.js', path: path.resolve(__dirname) } };
- To shim ES modules, you'll need to import the file directly. Update index.html to import the polyfill:
<!-- index.html --> <script type="module"> import './node_modules/babel-polyfill/dist/polyfill.min.js'; import { main } from './main.js'; main(); </script>
- Now open a browser, open the Developer Console, and visit the URL:
http://localhost:8000/. - Whether the browser supports Array.prototype.values or not, the code should run and display output as follows:
