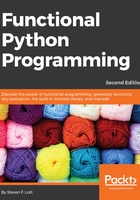
Using the map() function to apply a function to a collection
A scalar function maps values from a domain to a range. When we look at the math.sqrt() function, as an example, we're looking at a mapping from the float value, x, to another float value, y = sqrt(x), such that . The domain is limited to positive values. The mapping can be done via a calculation or table interpolation.
The map() function expresses a similar concept; it maps values from one collection to create another collection. It assures that the given function is used to map each individual item from the domain collection to the range collection-the ideal way to apply a built-in function to a collection of data.
Our first example involves parsing a block of text to get the sequence of numbers. Let's say we have the following chunk of text:
>>> text= """\ ... 2 3 5 7 11 13 17 19 23 29 ... 31 37 41 43 47 53 59 61 67 71 ... 73 79 83 89 97 101 103 107 109 113 ... 127 131 137 139 149 151 157 163 167 173 ... 179 181 191 193 197 199 211 223 227 229 ... """
We can restructure this text using the following generator function:
>>> data= list(
... v for line in text.splitlines()
... for v in line.split())
This will split the text into lines. For each line, it will split the line into space-delimited words and iterate through each of the resulting strings. The results look as follows:
['2', '3', '5', '7', '11', '13', '17', '19', '23', '29',
'31', '37', '41', '43', '47', '53', '59', '61', '67', '71',
'73', '79', '83', '89', '97', '101', '103', '107', '109', '113',
'127', '131', '137', '139', '149', '151', '157', '163', '167',
'173', '179', '181', '191', '193', '197', '199', '211', '223',
'227', '229']
We still need to apply the int() function to each of the string values. This is where the map() function excels. Take a look at the following code snippet:
>>> list(map(int, data)) [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59,
61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131,
137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197,
199, 211, 223, 227, 229]
The map() function applied the int() function to each value in the collection. The result is a sequence of numbers instead of a sequence of strings.
The map() function's results are iterable. The map() function can process any type of iterable.
The idea here is that any Python function can be applied to the items of a collection using the map() function. There are a lot of built-in functions that can be used in this map-processing context.