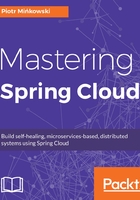
Introducing Spring Boot
Spring Boot is dedicated to running standalone Spring applications, the same as simple Java applications, with the java -jar command. The basic thing that makes Spring Boot different than standard Spring configuration is simplicity. This simplicity is closely related to the first important term we need to know about, which is a starter. A starter is an artifact that can be included in the project dependencies. It does nothing more than provide a set of dependencies to other artifacts that have to be included in your application in order to achieve the desired functionality. A package delivered in that way is ready for use, which means that we don't have to configure anything to make it work. And that brings us to the second important term related to Spring Boot, auto-configuration. All artifacts included by the starters have default settings set, which can be easily overridden using properties or other types of starters. For example, if you include spring-boot-starter-web in your application dependencies it embeds a default web container and starts it on the default port during application startup. Looking forward, the default web container in Spring Boot is Tomcat, which starts on port 8080. We can easily change this port by declaring the specified field in the application properties file and even change the web container by including spring-boot-starter-jetty or spring-boot-starter-undertow in our project dependencies.
Let me say a few words more about starters. Their official naming pattern is spring-boot-starter-*, where * is the particular type of starter. There are plenty of starters available within Spring Boot, but I would like to give you a short briefing on the most popular of them, which have also been used in the examples provided in the following chapters of this book:
Name | Description |
spring-boot-starter | Core starter, including auto-configuration support, logging, and YAML. |
spring-boot-starter-web | Allows us to build web applications, including RESTful and Spring MVC. Uses Tomcat as the default embedded container. |
spring-boot-starter-jetty | Includes Jetty in the project and sets it as the default embedded servlet container. |
spring-boot-starter-undertow | Includes Undertow in the project and sets it as the default embedded servlet container. |
spring-boot-starter-tomcat | Includes Tomcat as the embedded servlet container. The default servlet container starter used by spring-boot-starter-web. |
spring-boot-starter-actuator | Includes Spring Boot Actuator in the project, which provides features for monitoring and managing applications. |
spring-boot-starter-jdbc | Includes Spring JBDC with the Tomcat connection pool. The driver for the specific database should be provided by yourself. |
spring-boot-starter-data-jpa | Includes all artifacts needed for interaction with relational databases using JPA/Hibernate. |
spring-boot-starter-data-mongodb | Includes all artifacts needed for interaction with MongoDB and initializing a client connection to Mongo on localhost. |
spring-boot-starter-security | Includes Spring Security in the project and enables basic security for applications by default. |
spring-boot-starter-test | Allows the creation of unit tests using such libraries as JUnit, Hamcrest, and Mockito. |
spring-boot-starter-amqp | Includes Spring AMQP to the project and starts RabbitMQ as the default AMQP broker. |
If you are interested in the full list of available starters, refer to the Spring Boot specification. Now, let's get back to the main differences between Spring Boot and standard configuration with Spring Framework. Like I mentioned before we can include spring-boot-starter-web, which embeds a web container into our application. With standard Spring configuration, we do not embed a web container into the application, but deploy it as a WAR file on the web container. This is a key difference and one of the most important reasons that Spring Boot is used for creating applications deployed inside microservice architecture. One of the main features of microservices is independence from other microservices. In this case, it is clear that they should not share common resources, such as databases or web containers. Deploying many WAR files on one web container is an anti-pattern for microservices. Spring Boot is, therefore, the obvious choice.
Personally, I have used Spring Boot while developing many applications, not only when working in a microservice environment. If you try it instead of standard Spring Framework configuration, you will not want to go back. In support of that conclusion you can find an interesting diagram that illustrates the popularity of Java frameworks repositories on GitHub: http://redmonk.com/fryan/files/2017/06/java-tier1-relbar-20170622-logo.png. Let's take a closer look at how to develop applications with Spring Boot.