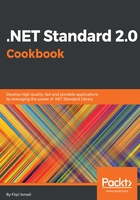
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open Visual Studio 2017.
- Now open the solution from the previous recipe. Click File | Open | Open Project/Solution, or press Ctrl + Shift + O, and select the Chapter2.Collections solution.
- Now, click on the Chapter2.Collections solution label. Click File | Add | New Project.
- In the Add New Project template dialog box, expand the Visual C# node in the left-hand pane.
- Select Windows Classic Desktop and then WPF App (.NET Framework) in the right-hand pane:

- In the Name: text box, type Chapter2.Collections.WPFLittleShop as the name of the project and leave the Location: text box as it is:

- Click OK.
- Now, the Solution Explorer (press Ctrl + Alt + L to open) should look like this:

- Now, double-click on the MainWindow.xaml to open the designer view.
- Open the Tool Box by pressing Ctrl + Alt + X and drag and drop two Buttons and two List Boxes to the main window.
- Place them as shown:

- Now, open the Properties window, or press F4, and change the following properties:
- After applying the previous properties, the MainWindow should look like this:

- Now, in the Solution Explorer (or press Ctrl + Alt + L), expand the Chapter2.Collections.WPFLittleShop project tree.
- Right-click on the References label and select Add Reference.
- In the Reference Manager dialog box, expand the Projects node in the left-hand pane.
- Click on Solution.
- Now, check Chapter2.Collections.CollectionsLib in the right-hand pane:

- Click OK.
- Double-click on the Get Fruits button.
- You will see the FruitsButton_Click() method.
- Scroll up the code window and add the following using directive at the end of all the using directives:
using Chapter2.Collections.CollectionsLib;
- Again, scroll down and inside the FruitsButton_Click() method, write the following code:
var littleShop = new LittleShop();
var fruits = littleShop.GetFruitsList();
foreach (var fruit in fruits)
{
FruitsList.Items.Add(fruit);
}
FruitsList.Items.Add("--------");
FruitsList.Items.Add($"Item Count: {fruits.Count}");
FruitsList.Items.Add($"Capacity: {fruits.Capacity}");
- Switch back to the MainWindow.xaml design view by clicking on its tab or just double-clicking on the MainWindow.xaml label in the Solution Explorer.
- Select the Get Items button and double-click on it.
- Now type the following code inside the ItemsButton_Click() event method:
var littleShop = new LittleShop();
var items = littleShop.GetShopItems();
for (int i=0; i<items.Count; i++)
{
ItemsList.Items.Add(items[i]);
}
ItemsList.Items.Add("--------");
ItemsList.Items.Add($"Item Count: {items.Count}");
ItemsList.Items.Add($"Capacity: {items.Capacity}");
- Let's press F5 and debug the code.
- Press the Get Fruits and Get Items buttons.
- You should see output like this:
