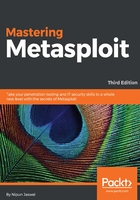
Loops in Ruby
Iterative statements are termed as loops; as with any other programming language, loops also exist in Ruby programming. Let's use them and see how their syntax differs from other languages:
def forl(a) for i in 0..a print("Number #{i}n") end end forl(10)
The preceding code iterates the loop from 0 to 10, as defined in the range, and consequently prints out the values. Here, we have used #{i} to print the value of the i variable in the print statement. The n keyword specifies a new line. Therefore, every time a variable is printed, it will occupy a new line.
Iterating loops through each loop is also a common practice and is widely used in Metasploit modules. Let's see an example:
def each_example(a) a.each do |i| print i.to_s + "t" end end # Main Starts Here a = Array.new(5) a=[10,20,30,40,50] each_example(a)
In the preceding code, we defined a method that accepts an array, a, and prints all its elements using the each loop. Performing a loop using the each method will store elements of the a array into i temporarily, until overwritten in the next loop. t, in the print statement, denotes a tab.
Refer to http://www.tutorialspoint.com/ruby/ruby_loops.htm for more on loops.