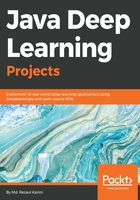
Preparing programming environment
In this section, we will discuss how to configure DL4J, ND4s, Spark, and ND4J before getting started with the coding. The following are prerequisites when working with DL4J:
- Java 1.8+ (64-bit only)
- Apache Maven for automated build and dependency manager
- IntelliJ IDEA or Eclipse IDE
- Git for version control and CI/CD
The following libraries can be integrated with DJ4J to enhance your JVM experience while developing your ML applications:
- DL4J: The core neural network framework, which comes up with many DL architectures and underlying functionalities.
- ND4J: Can be considered as the NumPy of the JVM. It comes with some basic operations of linear algebra. Examples are matrix creation, addition, and multiplication.
- DataVec: This library enables ETL operation while performing feature engineering.
- JavaCPP: This library acts as the bridge between Java and Native C++.
- Arbiter: This library provides basic evaluation functionalities for the DL algorithms.
- RL4J: Deep reinforcement learning for the JVM.
- ND4S: This is a scientific computing library, and it also supports n-dimensional arrays for JVM-based languages.
If you are using Maven on your preferred IDE, let's define the project properties to mention the versions in the pom.xml file:
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
<nd4j.version>1.0.0-alpha</nd4j.version>
<dl4j.version>1.0.0-alpha</dl4j.version>
<datavec.version>1.0.0-alpha</datavec.version>
<arbiter.version>1.0.0-alpha</arbiter.version>
<logback.version>1.2.3</logback.version>
<dl4j.spark.version>1.0.0-alpha_spark_2</dl4j.spark.version>
</properties>
Then use the following dependencies required for DL4J, ND4S, ND4J, and so on:
<dependencies>
<dependency>
<groupId>org.nd4j</groupId>
<artifactId>nd4j-native</artifactId>
<version>${nd4j.version}</version>
</dependency>
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>dl4j-spark_2.11</artifactId>
<version>1.0.0-alpha_spark_2</version>
</dependency>
<dependency>
<groupId>org.nd4j</groupId>
<artifactId>nd4j-native</artifactId>
<version>1.0.0-alpha</version>
<type>pom</type>
</dependency>
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>deeplearning4j-core</artifactId>
<version>${dl4j.version}</version>
</dependency>
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>deeplearning4j-nlp</artifactId>
<version>${dl4j.version}</version>
</dependency>
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>deeplearning4j-zoo</artifactId>
<version>${dl4j.version}</version>
</dependency>
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>arbiter-deeplearning4j</artifactId>
<version>${arbiter.version}</version>
</dependency>
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>arbiter-ui_2.11</artifactId>
<version>${arbiter.version}</version>
</dependency>
<dependency>
<artifactId>datavec-data-codec</artifactId>
<groupId>org.datavec</groupId>
<version>${datavec.version}</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.3.5</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>${logback.version}</version>
</dependency>
</dependencies>
By the way, DL4J comes with Spark 2.1.0. Additionally, if a native system BLAS is not configured on your machine, ND4J's performance will be reduced. You will experience the following warning once you execute simple code written in Scala:
****************************************************************
WARNING: COULD NOT LOAD NATIVE SYSTEM BLAS
ND4J performance WILL be reduced
****************************************************************
However, installing and configuring BLAS such as OpenBLAS or IntelMKL is not that difficult; you can invest some time and do it. Refer to the following URL for further details: http://nd4j.org/getstarted.html#open.
Well done! Our programming environment is ready for simple deep learning application development. Now it's time to get your hands dirty with some sample code.