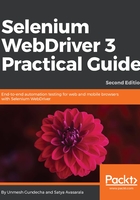
Setting preferences
Now we will learn how to set our own preferences. As an example, we will see how to change the user agent of your browser. These days, many web applications have a main site as well as a mobile site/m. site. The application will validate the user agent of the incoming request and decide whether to act as a server for a normal site or mobile site. So, in order to test your mobile site from your laptop or desktop browser, you just have to change your user agent. Let's see a code example where we can change the user-agent preference of our Firefox browser using FirefoxDriver, and send a request to the Facebook homepage. But before that, let's see the setPreference() method provided by the FirefoxProfile class:
public void setPreference(java.lang.String key, String value)
The input parameters are key, which is a string and represents your preference, and value, which has to be set to the preference.
There are two other overloaded versions of the preceding method shown; one of which is as follows:
public void setPreference(java.lang.String key, int value)
Here is the other overloaded version:
public void setPreference(java.lang.String key,boolean value)
Now, using the preceding setPreference() method, we will try to change the user agent of our browser using the following code:
public class SettingPreferences {
public static void main(String... args) {
System.setProperty("webdriver.gecko.driver",
"./src/test/resources/drivers/geckodriver 2");
FirefoxProfile profile = new FirefoxProfile();
profile.setPreference("general.useragent.override",
"Mozilla/5.0 (iPhone; CPU iPhone OS 11_0 like Mac OS X) " +
"AppleWebKit/604.1.38 (KHTML, like Gecko) Version/11.0 " +
"Mobile/15A356 Safari/604.1");
FirefoxOptions firefoxOptions = new FirefoxOptions();
firefoxOptions.setProfile(profile);
FirefoxDriver driver = new FirefoxDriver(firefoxOptions);
driver.get("http://facebook.com");
}
}
In the preceding code for the setPreference() method, general.useragent.override is set as the name of the preference, and the second parameter is the value for that preference, which represents the iPhone user agent. Now open the user.js file for this particular Firefox instance, and you will see the entry for this preference. You should use the following preference in your user.js file:
user_pref("general.useragent.override", "Mozilla/5.0 (iPhone; CPU iPhone OS 11_0 like Mac OS X) AppleWebKit/604.1.38 (KHTML, like Gecko) Version/11.0 Mobile/15A356 Safari/604.1");
Apart from this, you will observe that the mobile version of the Facebook homepage has been served to you.