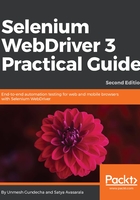
Understanding the Firefox profile
A Firefox profile is a folder that the Firefox browser uses to store all your passwords, bookmarks, settings, and other user data. A Firefox user can create any number of profiles with different custom settings and use it accordingly. According to Mozilla, the following are the different attributes that can be stored in the profiles:
- Bookmarks and browsing history
- Passwords
- Site-specific preferences
- Search engines
- A personal dictionary
- Autocomplete history
- Download history
- Cookies
- DOM Storage
- Security certificate settings
- Security device settings
- Download actions
- Plugin MIME types
- Stored sessions
- Toolbar customizations
- User styles
To create, rename, or delete a profile, you have to perform the following steps:
- Open the Firefox profile manager. To do that, in the command prompt terminal, navigate to the install directory of Firefox; typically, it is in Program Files if you are on Windows. Navigate to the location where you can find the firefox binary file, and execute the following command:
/path/to/firefox -p
It will open the profile manager, which will look like the following screenshot:
Note that before executing the preceding command, you need to make sure you close all your currently-running Firefox instances.
- Use the Create Profile... button to create another profile, the Rename Profile... button to rename an existing profile, and the Delete Profile... button to delete one.
So, coming back to our WebDriver, whenever we create an instance of FirefoxDriver, a temporary profile is created and used by the WebDriver. To see the profile that is currently being used by a Firefox instance, navigate to Help | Troubleshooting Information.
This will launch all the details of the particular Firefox instance of which the profile is a part. It will look similar to the following screenshot:
The highlighted oval in the preceding screenshot shows the profile folder. Click on the Show Folder button; it should open the location of the profile corresponding to that of your current Firefox instance. Now, let's launch a Firefox browser instance using our FirefoxDriver, and verify its profile location.
Let's launch a Firefox browser using the following code:
public class FirefoxProfile {
public static void main(String... args) {
System.setProperty("webdriver.gecko.driver",
"./src/test/resources/drivers/geckodriver 2");
FirefoxDriver driver = new FirefoxDriver();
driver.get("http://www.google.com");
}
}
This will launch a browser instance. Now, navigate to Help | Troubleshooting Information, and once the info is launched, click the Show Folder button. This will open the current WebDriver's profile directory. Every time you launch a Firefox instance using Firefox Driver, it will create a new profile for you. If you go one level above this directory, you will see the profiles created by your FirefoxDriver, as shown in the following screenshot:
All the preceding folders correspond to each of the Firefox instances launched by the FirefoxDriver.
So far, we have seen what Firefox profiles are and how WebDriver creates one every time it launches the browser. Now, let's see how we can create our own custom profiles using WebDriver APIs. The following is the code example to create your own Firefox profile using the WebDriver library and set the options you want your browser to have, overriding what FirefoxDriver gives you:
public class FirefoxCustomProfile {
public static void main(String... args) {
System.setProperty("webdriver.gecko.driver",
"./src/test/resources/drivers/geckodriver 2");
FirefoxProfile profile = new FirefoxProfile();
FirefoxOptions firefoxOptions = new FirefoxOptions();
firefoxOptions.setProfile(profile);
FirefoxDriver driver = new FirefoxDriver(firefoxOptions);
try {
driver.get("http://www.google.com");
} finally {
driver.quit();
}
}
}
In the preceding code, FirefoxProfile is the class that has been instantiated to create a custom profile for the Firefox browser launched from the test. Now, having an instance of that class, we can set various options and preferences in it, which we will discuss shortly. First, there are two overloaded versions of constructors for FirefoxProfile. One creates an empty profile and moulds it according to requirements. This is seen in the preceding code. The second version creates a profile instance from an existing profile directory, as follows:
public FirefoxProfile(java.io.File profileDir)
Here, the profileDir input parameter is the directory location of an existing profile. The profile directory is the one we saw in the preceding screenshot. Let's discuss some interesting customizations that we can do to our Firefox browser using Firefox profiles.