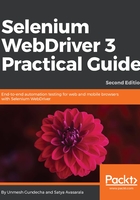
The By.xpath() method
WebDriver uses XPath to identify a WebElement on the web page. Before we see how it does that, let's quickly look at the syntax for XPath. XPath is a short name for the XML path, the query language used for searching XML documents. The HTML for our web page is also one form of the XML document. So, in order to identify an element on an HTML page, we need to use a specific XPath syntax:
- The root element is identified as //.
- To identify all the div elements, the syntax will be //div.
- To identify the link tags that are within the div element, the syntax will be //div/a.
- To identify all the elements with a tag, we use *. The syntax will be //div/*.
- To identify all the div elements that are at three levels down from the root, we can use //*/*/div.
- To identify specific elements, we use attribute values of those elements, such as //*/div/a[@id='attrValue'], which will return the anchor element. This element is at the third level from the root within a div element and has an id value of attrValue.
So, we need to pass the XPath expression to the By.xpath locating mechanism to make it identify our target element.
Now, let's see the code example and how WebDriver uses this XPath to identify the element:
@Test
public void byXPathLocatorExample() {
WebElement searchBox =
driver.findElement(By.xpath("//*[@id='search']"));
searchBox.sendKeys("Bags");
searchBox.submit();
assertThat(driver.getTitle())
.isEqualTo("Search results for: 'Bags'");
}
In the preceding code, we are using the By.xpath locating mechanism and passing the XPath of the WebElement to it.
One disadvantage of using XPath is that it is costly in terms of time. For every element to be identified, WebDriver actually scans through the entire page, which is very time consuming, and too much usage of XPath in your test script will actually make it too slow to execute.