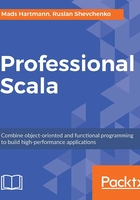
OO in Our Chatbot
Now that you know the theoretical basics, let's look at these facilities and how they are used in our program. Let's open Lesson 2/3-project
in our IDE and extend our chatbot, which was developed in the previous chapter.
Decoupling Logic and Environment
To do this, we must decouple the environment and logic, and integrate only one in the main
method.
Let's open the EffectsProvider
class:
Note
For full code, refer to Code Snippets/Lesson 2.scala
file.
trait EffectsProvider extends TimeProvider { def input: UserInput def output: UserOutput } object DefaultEffects extends EffectsProvider { override def input: UserInput = ConsoleInput override def output: UserOutput = ConsoleOutput override def currentTime(): LocalTime = LocalTime.now() override def currentDate(): LocalDate = LocalDate.now() }
Here, we encapsulate all of the effects into our traits, which can have different implementations.
For example, let's look at UserOutput
:
For full code, refer to Code Snippets/Lesson 2.scala
file.
trait UserOutput { def write(message: String): Unit def writeln(message: String): Unit = { write(message) write("\n") } } object ConsoleOutput extends UserOutput { def write(message: String): Unit = { Console.print(message) } }
Here, we can see the trait and object, which implement the current trait. This way, when we need to accept commands that are not from standard input, but from the chatbot API or from Twitter, we only need to change the implementation of the UserOutput
/ ConsoleOutput
interfaces.
It's now time to implement ConsoleOutput
and DefaultTimeProvider
.
Replace ???
in main with the appropriative constructor.
These steps for implementing ConsoleOutput
and DefaultTimeProvider
are as follows:
- Ensure that
Lesson 2/3-project
is open in IDE. - In the
UserOutput
file, find theConsoleOutput
file and change???
to the body of thewrite
method. The resulting method should look like this:object ConsoleOutput extends UserOutput{ def write(message: String): Unit = { Console.print(message) } } }
- In the
TimeProvider
file, add theDefaultTimeProvide
object which extends fromTimeProvider
and implements thecurrentTime
andcurrentDate
functions. The resulting code should look like this:object DefaultTimeProvider extends TimeProvider { override def currentTime(): LocalTime = LocalTime.now() override def currentDate(): LocalDate = LocalDate.now() } }