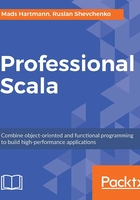
Unit Testing
In any program which is bigger than arithmetic operations, programmers should make themselves comfortable when it is possible to ensure that new changes are not breaking old functionalities.
The most common technique for this is unit testing, which is where the programmer tests the functionality of the code in parallel with its development by creating a test code which will verify that the code really satisfies their requirements.
The theme of this section will be introducing tools for unit testing in Scala.
Adding a Test to Our Project
Let's add tests to our small program. We'll import <for-students/lesson1/2-project>
in our IDE.
This is the directory schema of a Scala project. For adding tests, we should do the following:
- Add test dependencies to
build.sbt
- Write tests in the source test directory
For adding dependency, let's add the following line to our build.sbt
:
libraryDependencies += "org.scalatest" %% "scalatest" % "3.0.4" % "test"
It's an expression in Scala DSL (domain-specific language), which means that we should add scalatest
to our set of library dependencies. Operators %%
and %
are used for forming the name and classifier for published artifacts. You can refer to the sb
t
documentation for more detail: http://www.scala-sbt.org/1.x/docs/Library-Dependencies.html.
Before compilation, sbt
will download scalatest
from a publicly available repository (Maven central), and when running tests, it will add scalatest
to the classpath.
We will now run sbt
tests from the command line.
- In the command-line environment, navigate to the root of the project and select the following test:
Lesson 1/2-project
- If you are using a Unix/Linux machine and your code is situated in
courses/pactscala
of your homedirectory
, then run the following command:> cd ~/courses/packscala/Lesson 1/2-project
- Run the following command
:
> sbt test
- You will get the expected output, which will include the following strings:
[info] ExampleSpec: [info] - example test should pass [info] StepTest: [info] - step of unparded word must be interesting
We will now see how to run sbt
tests from IDEA IDE.
We'll now run sbt Tests from IDEA IDE.