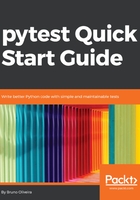
Output capturing: -s and --capture
Sometimes, developers leave print statements laying around by mistake, or even on purpose, to be used later for debugging. Some applications also may write to stdout or stderr as part of their normal operation or logging.
All that output would make understanding the test suite display much harder. For this reason, by default, pytest captures all output written to stdout and stderr automatically.
Consider this function to compute a hash of some text given to it that has some debugging code left on it:
import hashlib
def commit_hash(contents):
size = len(contents)
print('content size', size)
hash_contents = str(size) + '\0' + contents
result = hashlib.sha1(hash_contents.encode('UTF-8')).hexdigest()
print(result)
return result[:8]
We have a very simple test for it:
def test_commit_hash():
contents = 'some text contents for commit'
assert commit_hash(contents) == '0cf85793'
When executing this test, by default, you won't see the output of the print calls:
λ pytest tests\test_digest.py
======================== test session starts ========================
...
tests\test_digest.py . [100%]
===================== 1 passed in 0.03 seconds ======================
That's nice and clean.
But those print statements are there to help you understand and debug the code, which is why pytest will show the captured output if the test fails.
Let's change the contents of the hashed text but not the hash itself. Now, pytest will show the captured output in a separate section after the error traceback:
λ pytest tests\test_digest.py
======================== test session starts ========================
...
tests\test_digest.py F [100%]
============================= FAILURES ==============================
_________________________ test_commit_hash __________________________
def test_commit_hash():
contents = 'a new text emerges!'
> assert commit_hash(contents) == '0cf85793'
E AssertionError: assert '383aa486' == '0cf85793'
E - 383aa486
E + 0cf85793
tests\test_digest.py:15: AssertionError
----------------------- Captured stdout call ------------------------
content size 19
383aa48666ab84296a573d1f798fff3b0b176ae8
===================== 1 failed in 0.05 seconds ======================
Showing the captured output on failing tests is very handy when running tests locally, and even more so when running tests on CI.