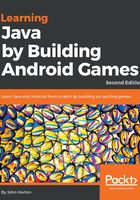
Understanding the Canvas class
The Canvas
class is part of the android.graphics
package. If you look at the import statements at the top of the Sub' Hunter code you will see these lines of code.
import android.graphics.Bitmap; import android.graphics.Canvas; import android.graphics.Color; import android.graphics.Paint; import android.graphics.Point; import android.view.Display; import android.widget.ImageView;
First, let's talk about Bitmap
, Canvas
, and ImageView
as highlighted in the previous code.
Getting started drawing with Bitmap, Canvas, and ImageView
As Android is designed to run on all types of mobile apps we can't immediately start typing our drawing code and expect it to work. We need to do a bit of preparation (coding) to get things working. It is true that some of this preparation can be slightly counterintuitive, but we will go through it a step at a time.
Canvas and Bitmap
Depending on how you use the Canvas
class, the term can be slightly misleading. While the Canvas
class is the class to which you draw your graphics, like a painting canvas, you still need a surface to transpose the canvas too.
The surface, in this game, will be from the Bitmap
class. We can think of it like this. We get a Canvas
object and a Bitmap
object and then set the Bitmap
object as the part of the Canvas
to draw upon.
This is slightly counterintuitive if you take the word canvas in its literal sense but once it is all set up we can forget about it and concentrate on the graphics we want to draw.
Tip
The Canvas class supplies the ability to draw. It has all the methods for doing things like drawing shapes, text, lines, image files and even plotting individual pixels.
The Bitmap
class is used by the Canvas class and is the surface that gets drawn upon. You can think of the Bitmap as being inside a picture frame on the Canvas.
Paint
In addition to Canvas
and Bitmap
, we will be using the Paint
class. This is much more easily understood. Paint
is the class used to configure specific properties like the color that we will draw on the Bitmap
within the Canvas
.
There is still another piece of the puzzle before we can get things drawn.
ImageView and Activity
The ImageView
is the class that the Activity
class will use to display output to the player. The reason for this third layer of abstraction is that not every app is a game (or graphics app). Therefore, Android uses the concept of "views" via the View
class to handle the final display the user sees.
There are multiple types of view enabling all different types of app to be made and they will all be compatible with the Activity
class that is the foundation of all regular Android apps and games.
It is, therefore, necessary to associate the Bitmap
which gets drawn on (through its association with Canvas
) with the ImageView
, once the drawing is done. The last step will be telling the Activity
that our ImageView
represents the content for the user to see.
Canvas, Bitmap, Paint and ImageView quick summary
If the theory of the interrelationship we need to set up seems like it is not simple you should breathe a sigh of relief when you see the relatively simple code very shortly.
A quick summary so far:
- Every app needs an
Activity
to interact with the user and the underlying operating system. Therefore, we must conform to the required hierarchy if we want to succeed. - The
ImageView
class, which is a type ofView
class is whatActivity
needs to display our game to the player. Throughout the book, we will use different types of theView
class to suit the project at hand. - The
Canvas
class supplies the ability to draw. It has all the methods for doing things like drawing shapes, text, lines, image files and even plotting individual pixels. - The
Bitmap
class is associated with theCanvas
class and it is the surface that gets drawn upon. - The
Canvas
class uses thePaint
class to configure details like the color. - Finally, once the Bitmap has been drawn upon we must associate it with the
ImageView
which in turn is set as the view for theActivity
The result is that what we drew on the Bitmap
with Canvas
that is displayed to the player through the ImageView
. Phew!
Tip
It doesn't matter if that isn't 100% clear. It is not you that isn't seeing things clearly- it simply isn't a clear relationship. Writing the code and using the techniques over and over will cause things to become clearer. Look at the code, do the demo app and then re-read this section.
Let's look at how to set up this relationship in code. Don't worry about typing the code, just study it. We will also do a hands-on drawing mini-app before we go back to the Sub' Hunter game.