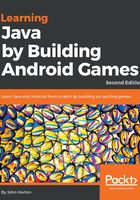
Printing Debugging Information
We can output all our debugging information and a good time to do this is in the draw
method because every time something happens the draw
method is called. But we don't want to clutter the draw
method with loads of debugging code. So, at the end of the draw
method add this call to printDebuggingText
.
/*
Here we will do all the drawing.
The grid lines, the HUD, and
the touch indicator.
*/
void draw() {
Log.d("Debugging", "In draw");
printDebuggingText();
}
Notice that the method is always called. You might ask what happens if debugging
is set to false
? We will amend this code when we have learned about making decisions in Chapter 7, Making Decisions with Java If, Else and Switch. We will also upgrade this next code to output the text in real-time to the device screen instead of the logcat window.
Now add this code to printDebuggingText
to output all the values to logcat.
// This code prints the debugging text public void printDebuggingText(){ Log.d("numberHorizontalPixels", "" + numberHorizontalPixels); Log.d("numberVerticalPixels", "" + numberVerticalPixels); Log.d("blockSize", "" + blockSize); Log.d("gridWidth", "" + gridWidth); Log.d("gridHeight", "" + gridHeight); Log.d("horizontalTouched", "" + horizontalTouched); Log.d("verticalTouched", "" + verticalTouched); Log.d("subHorizontalPosition", "" + subHorizontalPosition); Log.d("subVerticalPosition", "" + subVerticalPosition); Log.d("hit", "" + hit); Log.d("shotsTaken", "" + shotsTaken); Log.d("debugging", "" + debugging); Log.d("distanceFromSub", "" + distanceFromSub); }
Although there is lots of code in the previous snippet it is all quickly explained. We are using Log.d
to write to the logcat as we have done before. What is new is that for the second part of the output we write things like "" + distanceFromSub
. This has the effect of concatenating the value held in an empty String
(nothing) with the value held in distanceFromSub
.
Examine the previous code once more and you can see that every line of code states the variable name inside the speech marks having the effect of printing the variable name. On each line of code, this is followed by the empty speech marks and variable concatenation which outputs the value of the variable.
Seeing the output will make things clear.