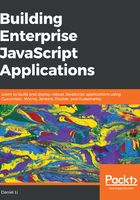
Making failure more informative
At the moment, if one of the assertions fails, we're throwing a generic Error object:
throw new Error();
When the test actually fails, the error message is not helpful because it doesn't tell us what the actual result is:
? Then our API should respond with a 400 HTTP status code
{}
Error
at World.<anonymous>
We can improve this by throwing an instance of AssertionError instead of just an instance of Error. AssertionError is a class provided by Node.js that allows you to specify the expected and actual outcomes.
To use it, first import it from the assert module:
import { AssertionError } from 'assert';
Then, change our step definition to the following:
Then('our API should respond with a 400 HTTP status code', function () {
if (this.response.statusCode !== 400) {
throw new AssertionError({
expected: 400,
actual: this.response.statusCode,
});
}
});
Now, when there's an error, the error output is much more informative:

However, we can go one better and use the equal method from the assert module directly. Now, our step definition is much more concise:
import assert from 'assert';
...
Then('our API should respond with a 400 HTTP status code', function () {
assert.equal(this.response.statusCode, 400);
assert.equal will automatically throw an AssertionError if the parameters passed into it are not equal.
Now do the same for the step definition that checks for the response's message:
Then('contains a message property which says "Payload should not be empty"', function () {
assert.equal(this.responsePayload.message, 'Payload should not be empty');
});