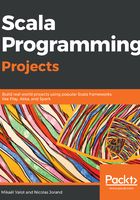
If...else expression
In Scala, if (condition) ifExpr else if ifExpr2 else elseExpr is an expression, and has a type. If all sub-expressions have a type A, the type of the if ... else expression will be A as well:
scala> def agePeriod(age: Int): String = {
if (age >= 65)
"elderly"
else if (age >= 40 && age < 65)
"middle aged"
else if (age >= 18 && age < 40)
"young adult"
else
"child"
}
agePeriod: (age: Int)String
If sub-expressions have different types, the compiler will infer a common super-type, or widen the type if it is a numeric type:
scala> val ifElseWiden = if (true) 2: Int else 2.0: Double
ifElseWiden: Double = 2.0
scala> val ifElseSupertype = if (true) 2 else "2"
ifElseSupertype: Any = 2
In the first expression present in the preceding code, the first sub-expression is of type Int and the second is of type Double. The type of ifElseWiden is widened to be Double.
In the second expression, the type of ifElseSupertype is Any, which is the common super-type for Int and String.
An if without an else is equivalent to if (condition) ifExpr else (). It is better to always specify the else expression, otherwise, the type of the if/else expression might not be the one we expect:
scala> val ifWithoutElse = if (true) 2
ifWithoutElse: AnyVal = 2
scala> val ifWithoutElseExpanded = if (true) 2: Int else (): Unit
ifWithoutElseExpanded: AnyVal = 2
scala> def sideEffectingFunction(): Unit = if (true) println("hello world")
sideEffectingFunction: ()Unit
In the preceding code, the common super-type between Int and Unit is AnyVal. This can be a bit surprising. In most situations, you would want to avoid that.