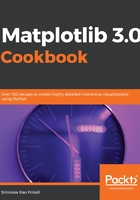
上QQ阅读APP看书,第一时间看更新
There's more...
Let's take one more example of how the logarithmic scale can be used. Let's say that within an organization, employees are classified into five categories based on their position. Each of these five categories is further split into men and women. For each of these 10 categories (five men and five women), we have the average salary and the standard deviation. This data is plotted to see how salary data is distributed across groups of men and women.
Here is the code and how it works:
- Import the required libraries:
import matplotlib.pyplot as plt
import numpy as np
- Define Python lists containing mean and standard deviation data for men and women:
menMeans = [3, 10, 100, 500, 50]
menStd = [0.75, 2.5, 25, 125, 12.5]
womenMeans = [1000, 3, 30, 800, 1]
womenStd = [500, 0.75, 8, 200, 0.25]
- Define the figure layout, the index list where the menMeans bars are to be placed on the x axis, and the width of each bar, and then plot the bar graph for men data in red with a black line on the head of the bar, indicating the size of the standard deviation for the group:
fig, ax = plt.subplots()
ind = np.arange(len(menMeans))
width = 0.35
p1 = ax.bar(ind, menMeans, width, color='lightblue', bottom=0,
yerr=menStd)
- Similarly, plot the womenMeans bar chart in yellow, adjacent to the menMeans bars, then set the title, xticks placement on the x axis, and their labels:
p2 = ax.bar(ind + width, womenMeans, width, color='orange',
bottom=0, yerr=womenStd)
ax.set_title('Scores by category and gender')
ax.set_xticks(ind + width / 2)
ax.set_xticklabels(('C1', 'C2', 'C3', 'C4', 'C5'))
- Finally, ax.set_yscale('log') sets the y axis scale to logarithmic and ax.legend((p1[0], p2[0]), ('Men', 'Women')) sets the legend for the plot. Then, plt.show() displays the plot on the screen.
Here is how the output bar plot looks:

Matplotlib supports four different scales. The default is linear, and we have covered log in this recipe . You can refer to the Matplotlib documentation for the other two scales: symlog and logit ( https://matplotlib.org/examples/scales/scales.html).