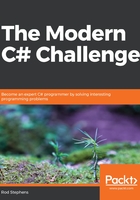
1. Statistical functions
Create a StatisticsExtensions class that defines extension methods to calculate statistical functions for arrays or lists of numbers. LINQ provides the Average, Max, and Min extension methods to calculate some statistical functions, so you don't need to implement those.
The following list summarizes the statistical functions that you should provide:
- Truncated mean: This is the mean (average) after removing an indicated number or percentage of the largest and smallest values. For example, if the values are {1, 1, 3, 5, 7, 7, 9} and you want to remove the two largest and smallest values, the remaining values are {3, 5, 7}.
- Median: This is the middlemost value. For example, if the values are {1, 1, 3, 5, 7, 7, 9}, then the median is 5 because half of the values are less than 5 and half are greater. If the set includes an even number of values, the median is the average of the two middlemost values.
- Mode: This is the value that occurs most often. In the set {1, 2, 3, 3, 7}, the mode is 3 because it appears twice. If there's a tie, return all of the modes in a list.
- Sample standard deviation: This is a measure of how widely spread the values are. The sample standard deviation is defined by the following formula:

- Population standard deviation: This is similar to the sample standard deviation except you divide by N instead of N – 1 in the equation.
In the standard deviation equation:
- The lowercase Greek sigma, σ, represents the standard deviation
- N is the number of items in the set
- The uppercase Greek sigma, ∑, means to add up the values to its right (in this case, the sums of the squares of the differences between the xi values and μ) as i ranges from 1 to N
- The lowercase Greek mu, μ, is the mean (average) of the values
Write a program similar to the one shown in the following screenshot to test your extension methods. This program generates the indicated number of values and displays statistics about them. Each value is the sum of two random values between 1 and 6, so the values give a bell curve. (The shape is more obvious if you generate more than 100 values.):
The example solution uses labels to build the histogram, showing the numbers' frequencies.