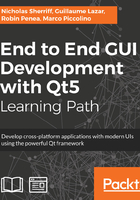
Projects, MVC, and unit testing
The scratchpad application we built in the previous chapter is a Qt project, represented by a .pro file. In a business environment, technical solutions are generally developed as part of company initiatives, and these initiatives are generally also called projects. To try and minimize confusion (and the number of times the word project appears!), we’ll use project to mean a Qt project defined by a .pro file and the word initiative to refer to projects in the business sense.
The initiative we will work on will be a generic client management system. It will be something that can be tweaked and re purposed for multiple applications—for a supplier managing customers, a health service managing patients, and so on. It will perform common tasks found over and over in real-world Line of Business (LOB) applications, principally adding, editing, and deleting data.
Our scratchpad application is entirely encapsulated within a single project. For smaller applications, this is perfectly viable. However, with larger code bases, particularly with several developers involved, it often pays to break things up into more manageable pieces.
We will be using a super lightweight implementation of the Model View Controller (MVC) architectural pattern. If you haven’t come across MVC before, it is primarily used to decouple business logic from the user interface. The user interface (View) relays commands to a switchboard style class (Controller) to retrieve the data and perform actions it needs. The controller in turn delegates the responsibility for the data, logic, and rules to data objects (Models):

The key is that the View knows about the Controller and the Model, as it needs to send commands to the Controller and display the data held in the Model. The Controller knows about the Model as it needs to delegate work to it, but it doesn’t know about the View. The Model knows nothing about either the Controller or the View.
A key benefit of designing the application this way in a business environment is that dedicated UX specialists can work on the views while programmers work on the business logic. A secondary boon is that because the business logic layer knows nothing about the UI, you add, edit, and even totally replace user interfaces without affecting the logic layer. A great use case would be to have a “full fat” UI for a desktop application and a companion “half fat” UI for a mobile device, both of which can use the same business logic. With all this in mind, we will physically segregate our UI and business logic into separate projects.
We will also look at integrating automated unit tests into our solution. Unit testing and Test Driven Development (TDD) has really grown in popularity in recent times and when developing applications in a business environment, you will more than likely be expected to write unit tests alongside your code. If not, you should really propose doing it as it holds a lot of value. Don’t worry if you haven’t done any unit testing before; it’s very straightforward, and we’ll discuss it in more detail later in the book.
Finally, we need a way to aggregate these subprojects together so that we don’t have to open them individually. We will achieve this with an umbrella solution project that does nothing other than tying the other projects together. This is how we will lay out our projects:
