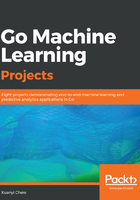
Packages and imports
Finally, we come to the concept of packages and imports. For the majority of the book, the projects described live in something called a main package. The main package is a special package. Compiling a main package will yield an executable file that you can run.
Having said that, it's also often a good idea to organize your code into multiple packages. Packages are a form of abstraction barrier that we discussed previously with regards to variables and names. Exported names are accessible from outside the package. Exported fields of structs are also accessible from outside the package.
To import a package, you need to invoke an import statement at the top of the file:
package main
import "PACKAGE LOCATION"
Throughout this book I will be explicit in what to import, especially with external libraries that cannot be found in the Go standard library. We will be using a number of those, so I will be explicit.
Go enforces code hygiene. If you import a package and don't use it, your program will not compile. Again, this is a good thing as it makes it less likely to confuse yourself at a later point in time. I personally use a tool called goimports to manage my imports for me. Upon saving my file, goimports adds the import statements for me, and removes any unused packages from my import statements.
To install goimports, run the following command in your Terminal:
go get golang.org/x/tools/cmd/goimports